Kruskal's Algorithm
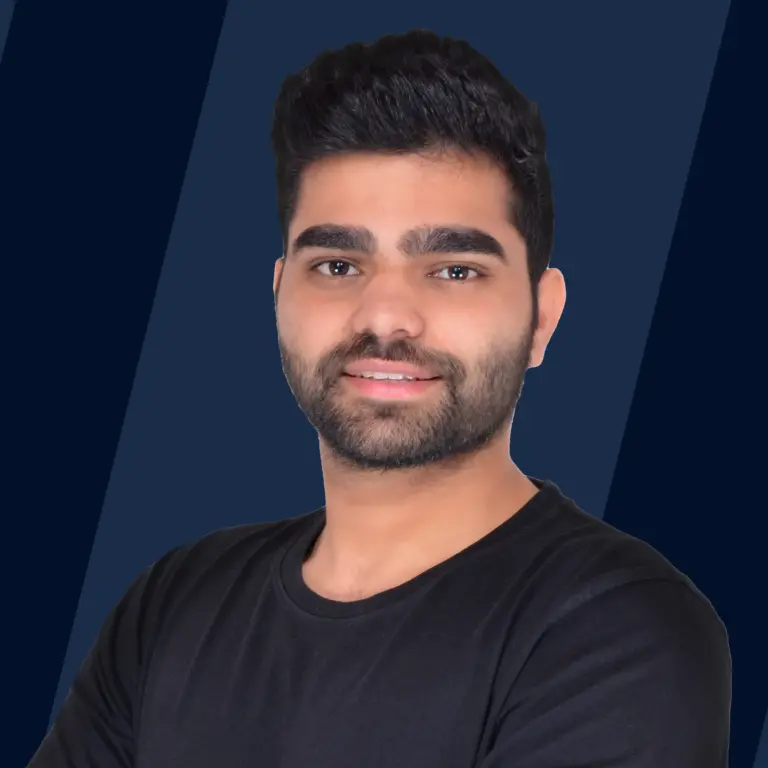
Overview
Kruskal's algorithm is a greedy algorithm in graph theory that is used to find the Minimum spanning tree (A subgraph of a graph which is a tree and includes all the vertices of the given graph such that the sum of the weight of the edges is minimum) of a given connected, weighted, undirected graph.
In case, the graph is not connected, on applying Kruskal's algorithm we can find the MST of each connected component.
Takeaways
Complexities of the Kruskal's Algorithm
- Time Complexity :
- Space Complexity:
Introduction to Kruskal’s Algorithm
Suppose that there are some villages in a town, you being the Mayor of the town want to visit all the villages but you have very little time for it.
So you will be interested in visiting the villages in such a way that the total distance covered by you during the visit is minimum possible. Isn't it.?
If you try to formulate the above problem in terms of graph theory, by considering villages as the vertices, roads connecting them as the edges, and the distance of each road as the weight of the corresponding edge.
Kurskal's algorithm will help you in this case because it is used to find the minimum spanning tree (MST) of any given connected, weighted, undirected graph. Before discussing any further details of Kruskal's algorithm let's see what is meant by a Minimum spanning tree of a graph.
A spanning tree is a subgraph of a connected, undirected graph such that it is a tree and it includes all the vertices. So a minimum spanning tree would correspond to a spanning tree with the minimum weight. Where the weight of a spanning tree is the sum of the weight of the edges present in it.
For Example:
The below-given image shows a graph with 6 vertices and 9 edges
This graph can have multiple spanning tree some of which are shown below with their respective weights.
But they can not be considered as minimum spanning trees as there exists at least one spanning tree with an even smaller sum of edge weights.
The MST of the graph is -
The MST of the given graph has a weight of 17, which means that we can't form any other spanning tree of graph whose weight is less than 17.
Kruskal's Algorithm Implementation
The main idea of Kruskal's algorithm to find the MST of a graph with vertices and edges is
- to sort all the edges in ascending order according to their weights.
- Then select the first edges such that they do not form any cycle within them.
One thing that can be observed here is, that for any graph with vertices, we are interested in including only edges as part of the MST. It is because we need only that many edges to include all the vertices.
Let us understand how Kruskal's Algorithm works by the following algorithm and example
Algorithm of Kruskal's
For any graph, , finding an MST using Kruskal's algorithm involves the following steps -
- Sort all the edges of the graph in ascending order of their weights.
- Check the edge with minimum weight, if including it in the answer forms a cycle discard it, otherwise include it in the answer.
- Repeat the above step until we have chosen edges.
Example of Kruskal's Algorithm
Let's say we want to find the MST of the underlying connected, weighted, undirected graph with vertices and edges-
Now as per the algorithm discussed above, to find the MST of this graph -
- We will write all the edges sorted in ascending order according to their respective weights
- Then we will select the first edges which do not form cycles.
-
Step 1 - Sort the edges in ascending order. Here is the list after sorting.
No. Weight 1 4 5 2 2 4 6 2 3 3 4 3 4 2 3 3 5 3 5 4 6 5 6 5 7 2 5 6 8 1 2 7 9 1 3 8 -
Step 2 - Choose 5 edges from starting which do not form a cycle.
-
Checking edge - This is the first edge so it can't form any cycle hence including this in result -
-
Checking for edge - Including this edge in the result does not form any cycle.
-
Checking for edge - Again including this edge in the result does not form any cycle.
-
Checking for edge - Including this edge in the result forms a cycle , hence not including this in the result.
-
Checking for edge - Including this edge in the result forms a cycle , hence not including this in the result.
-
Checking for edge - Including this edge in the result forms a cycle , hence not including this in the result.
-
Checking for edge - Including this edge in the result does not form any cycle. By including this, we have included 5 edges so now the result will correspond to the minimum spanning tree.
-
After including all edges, the MST will look like this -
So the weight of MST can be calculated as .
Pseudocode of Kruskals Algorithm
Code Example of C/C++, Python
To efficiently check whether including an edge forms a cycle or not, we will use an already discussed concept Disjoint-Sets. We will proceed as per the pseudocode and algorithm discussed above, firstly sorting the list of edges and then including edges that do not form cycles. Find function of DSU will be used before including any edge in the MST to check if both the endpoints (nodes) of the edge belong to the same set or not. If they do not belong to the same set, we will include that edge in the MST as including the edge is not forming any cycle. Now we will use the union function of DSU to merge the two disjoint sets. Before going into the code, let's see its blueprint -
Variables -
- - A global variable that will denote the number of vertices present in the graph.
- - Again a global variable denoting the count of edges present in the graph.
- - A list of edges that will be sorted and then used in the Kruskal algorithm.
Method -
- - A function that is responsible to find the MST of a given graph implemented as discussed in the algorithm and pseudocode.
Future-Proof Your Coding Skills! Join Our Best DSA Course for In-Depth Algorithmic Understanding. Enroll Now!
C/C++ Implementation of Kruskal's Algorithm
Java Implementation of Kruskal's Algorithm
Complexity Analysis
- Time Complexity -
- Sorting of edges costs us time.
- For each edge, we are using the Union-Find algorithm which costs us time.
- As discussed in DSU, for practical values of , we can write as because .
- Space Complexity - We are using a List/Vector to store the edges of the given graph so the space complexity is .
Applications of Kruskal's Algorithm
MST's which can be found using the Kruskal algorithm has the following applications -
- In-network designing, finding MST tells you the minimum amount of wire needed to connect all the nodes (servers).
- Approximation of NP-Hard problems, like we can use MST to solve the traveling salesman problem.
- It is used in autoconfig protocol for ethernet bridging, which helps to avoid cycles in a network.
- All other graph theory problems where we need to visit all the vertices with minimum cost.
Become a master of data structures with our Data Structures in C++ Free Course. Start your journey today!
Conclusion
- In this article, we have learned what is meant by the spanning trees of a graph and what is the minimum spanning tree with the help of examples.
- We have seen Kruskal's algorithm which is a greedy algorithm to find an MST of any given weighted, undirected graph.
- At last, we have analyzed its time and complexity and space complexity and seen some of its major applications.