dir() in Python
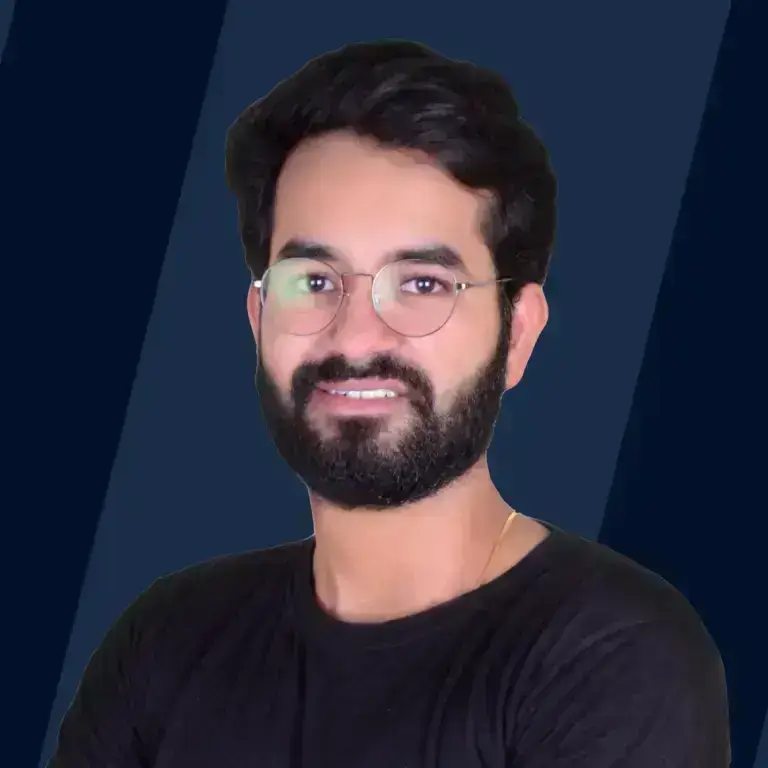
Overview
The dir() function in python is an in-built function used on an object to look at all the properties / attributes and methods of that object, without its values (if the values of the attributes are given).
Syntax of dir() in Python
The python dir function, or "directory" is used in the following way:
Parameters of dir() in Python
The dir function in python takes only one parameter:
- object(optional): i.e. we are not required to pass any parameters to know all the defined names in the namespace. However, to know the attributes / properties of a particular object you must pass it as a parameter.
This object could be a function, or a module, lists, strings or even dictionaries etc.
Return Values of dir() in Python
dir() in Python returns all the defined names in a namespace as a list. For different python objects, the dir function in Python gives different results.
- If the input object is a class, a list of names containing all the valid attributes along with base attributes is returned.
- If the input object are modules or library objects, the list of names of all attributes that are contained in that module is returned.
- However, if no parameters are given to the function, it simply returns all the names in the current local scope, of course in the form of a list.
What is the dir() function in Python?
The dir() function in python is one of the very powerful built-in functions. It is essentially short for "directory", which does exactly as its name, provides us with all the names in the current local scope in the form of a list.
Applications of dir() in Python
The dir() function in python is usually used for debugging purposes, in day to day programming. The functionality of being able to return all attributes of the object passed to it as a parameter in a list is useful when dealing with a plethora of classes and functions.
This function is also particularly helpful when you're working with a new module that you created, or even an existing one and would like to know about all the available attributes and functions. For example, you are working on mathematical equations and might want to know if the 'math' module that you are working with, contains a function to calculate the gcd (greatest common divisor) of two numbers. You can simply call the dir() in python and list out all the available functions in the current namespace of the module as well as the inbuilt functions.
Now that you know what the dir() function does, let's take a look at some examples on how you can make use of it in your code.
Examples of dir() in python
Let's first call the dir() function without any parameters.
Code:
Output:
What if you imported some modules in your code, what would the dir() function return without any parameters?
Code:
Output:
As you can see, two new names have been added to the dir() function output, and they are the two modules that we imported. In both the outputs, these are the basic built-in functions that are available to us.
Now let's try finding out all the functions avaiable to us in the math module.
Code:
Output:
As you can see in the output, the dir() function has returned a list of all the avaiable functions present in the math module. What if you also added a list in your local scope?
Code:
Output:
Explanation:
You can see that the new object that we added to our local scope, our list has been added to the output of the dir() function. We read earlier that the dir() function is not only used on modules and libraries but also on basic objects like dictionaries. We're going to pass two objects as parameters to the dir() function, a dictionary and a list. It should return all the available list and dictionary functions in the current local scope.
Code:
Output:
As you can see, the dir() function has returned all the avaiable methods as a list. Let's now try creating an object of a class we created. We're going to take the example of a 'Player' in a very common game - Monopoly / Business.
Code:
Output:
As you can see, the dir() function returned a list of all the attributes of the Player() class.
This simple dir() function in python is used mostly for debugging purposes by developers for small and large projects. It comes in handy when there are a lot of classes and functions being handled separately. And voila, you now know all there is to the dir function in python.
Also, check this article on how to Create Directory in Python.
Conclusion
- The dir() function in python is an inbuilt function used on an object to look at all the properties / attributes and methods of that object, without its values (if any).
- It is used as dir({object}) and takes only one optional parameter - the object.
- Returns all the defined names in a namespace in the form of a list.