Python List reverse()
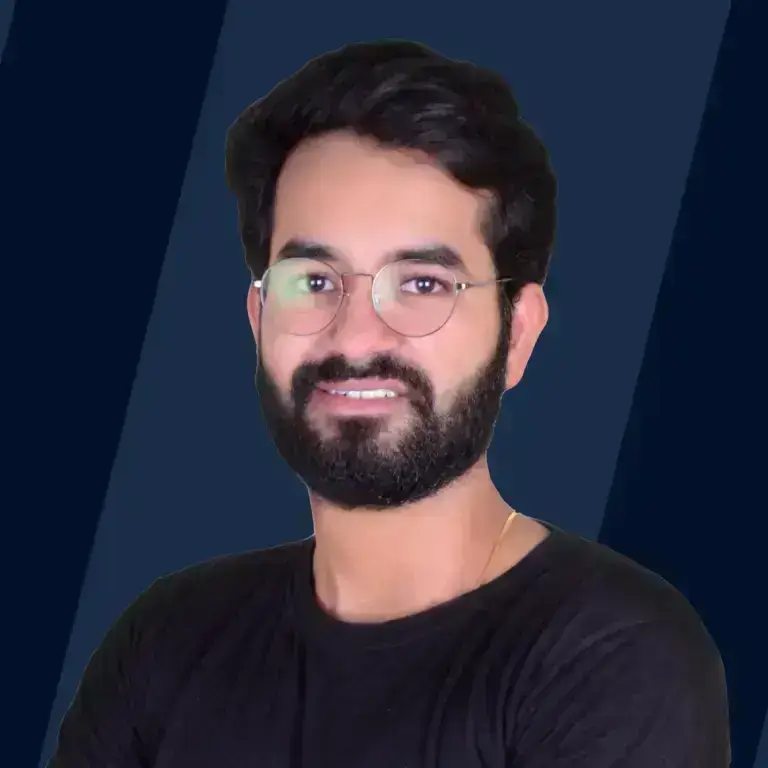
Reversing a list is a common operation in Python programming, allowing you to invert the order of elements in a sequence. This can be achieved effortlessly using Python's built-in methods.
Example:
Output:
How to Reverse a List in Python
Python offers various methods to reverse a list, each catering to different scenarios and preferences. Below, we explore six effective ways to achieve this:
Using Slicing Technique
The slicing technique in Python allows you to reverse a list by specifying the start, stop, and step parameters in slice notation list[::-1]. This method creates a new reversed list.
Example 1:
Output: [5, 4, 3, 2, 1]
Example 2:
Output: ['d', 'c', 'b', 'a']
Time Complexity: , as it creates a new list with reversed elements.
Space Complexity: , due to the creation of a new list.
Reversing a List in Python Using Swapping Present and Last Numbers
This method involves manually swapping elements in the list. You iterate over the list, exchanging the current element with the corresponding element from the end, moving towards the middle. This technique effectively reverses the list in place.
Based on the structure and logic of the provided function, here's an alternative example that demonstrates the same concept of reversing a list using swapping:
Output: [50, 40, 30, 20, 10]
Time Complexity:
Space Complexity:
Using reversed() and reverse() Built-in Python Functions
The reversed() function returns an iterator that accesses the given sequence in the reverse order. The reverse() method directly modifies the original list.
Example 1: with reversed function:
Output: 5 4 3 2 1
Example 2: with reverse function in python:
Output: ['d', 'c', 'b', 'a']
Time Complexity: , reverses the list in place.
Space Complexity: , as no additional space is used.
Using insert() Function
The insert() function can be used to insert each element at the beginning of a new list, effectively reversing the order.
Example 1:
Output: [5, 4, 3, 2, 1]
Example 2:
Output: ['world', 'hello']
Time Complexity:
Space Complexity:
Using List Comprehension
List comprehension provides a concise way to reverse a list by iterating over the original list in reverse order.
Example 1:
Output: [5, 4, 3, 2, 1]
Example 2:
Output: ['d', 'c', 'b', 'a']
Time Complexity:
Space Complexity:
Using NumPy
NumPy, a powerful library for numerical operations, can be used to reverse an array efficiently. This method is particularly useful for large datasets.
Example 1:
Output: [5 4 3 2 1]
Example 2:
Output: ['cherry' 'banana' 'apple']
Time Complexity:
Space Complexity:
Conclusion
- There are many ways to reverse the list.
- We can use any of the methods depending on the type of need.
- reverse() method reverses the list without creating a new copy but modifies the original list.
- reversed() function returns an iterator that iterates the list in reverse order.
- Slicing technique reverses the list without modifying the original list but takes extra space.