Reversed in Python | Python reversed() Function
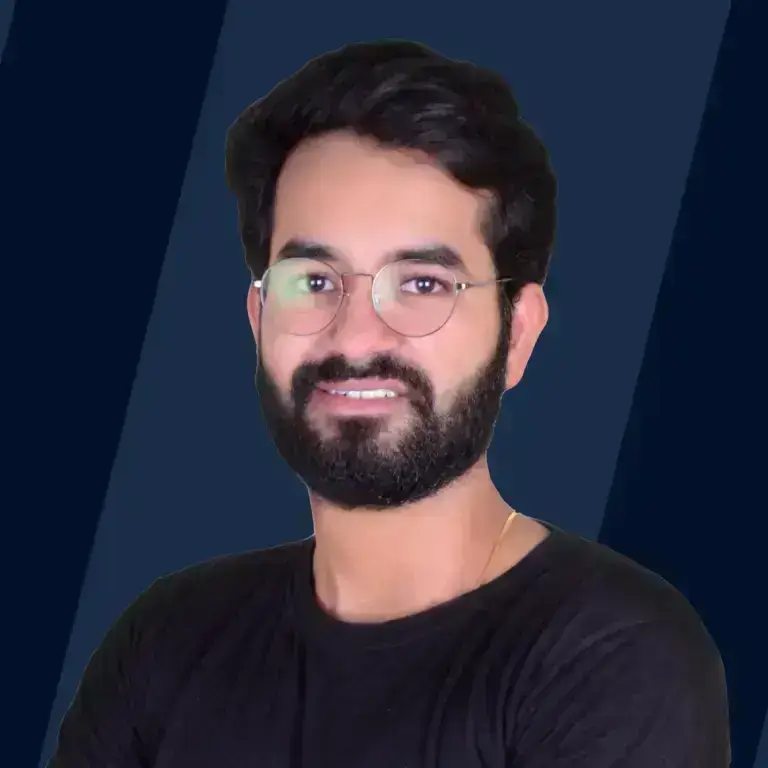
Overview
The built-in reversed function in Python is used to reverse a sequence like a list, string, or tuple by creating a reverse iterator of the given sequence.
Syntax of reversed() in Python
The syntax of reversed function in Python is:
Parameters of reversed() in Python
The reversed function takes a single parameter:
- seq: the name of the sequence (list, str, tuple) whose reverse iterator is needed.
Return Values of reversed() in Python
Return Type: <class 'reversed'>
reversed in Python returns an iterator that accesses the given sequence in the reverse order.
Exceptions of reversed() in Python
- StopIteration: The reversed function in Python will raise StopIteration error if we call next method to access an element even after getting the last element.
Example
Output of reversed in Python
What is Reversed in Python?
reversed is a built-in function in Python used to get a reversed iterator of a sequence.
reversed function is similar to the iter() method but in reverse order.
An iterator is an object used to iterate over an iterable.
- We can generate an iterator object using the iter method on an iterable.
- Use the next function to get a value from an iterable using an iterator.
Example:
Output:
If you aren't familiar with iterators, you can go through this article on Scaler Topics: Iterators in Python.
We can use the reversed function with any sequence or class that implements the __reverse()__ method.
More Examples
Example 1: Using reversed() in string, tuple, list, and range
Output:
Explanation:
We used the reversed function with a list, string, tuple, and a range object. As we can't see the elements inside an iterator object directly, we need to convert the return value to either a list or tuple so that we can see the output. Printing a reversed iterator is not meaningful.
Example 2: reversed() with for loop
Output:
Explanation:
We can use the reversed function with a for a loop because it returns an iterator. In this example, we used the reversed function to get the reverse of my_string then we used it inside a for loop to print each character one by one.
Example 3: reversed() in custom objects()
Output:
Explanation:
As stated before, we can use reversed with any class that implements the __reversed__ method.
Example 4: Example of Exception in reversed() function
Output:
Explanation:
We can use the next function to access a value from an iterator. Once the iterator is exhausted, the next function will raise a StopIteration error.
In this example, we reversed a list with three elements and then accessed each element with the next function after accessing the 1, the last element in the reversed list. The iterator gets exhausted. That's why using the next function in the fourth print statement raises the StopIteration error.
Conclusion
- The built-in reversed function in Python is used to reverse a sequence.
- Reversed function in Python takes a sequence as an argument and returns a reverse iterator over the elements.
- We can use the reversed function with any sequence or class that implements the reverse() method.
- The reversed function in Python raises a StopIteration error when all the elements are exhausted.