Java 8 Features
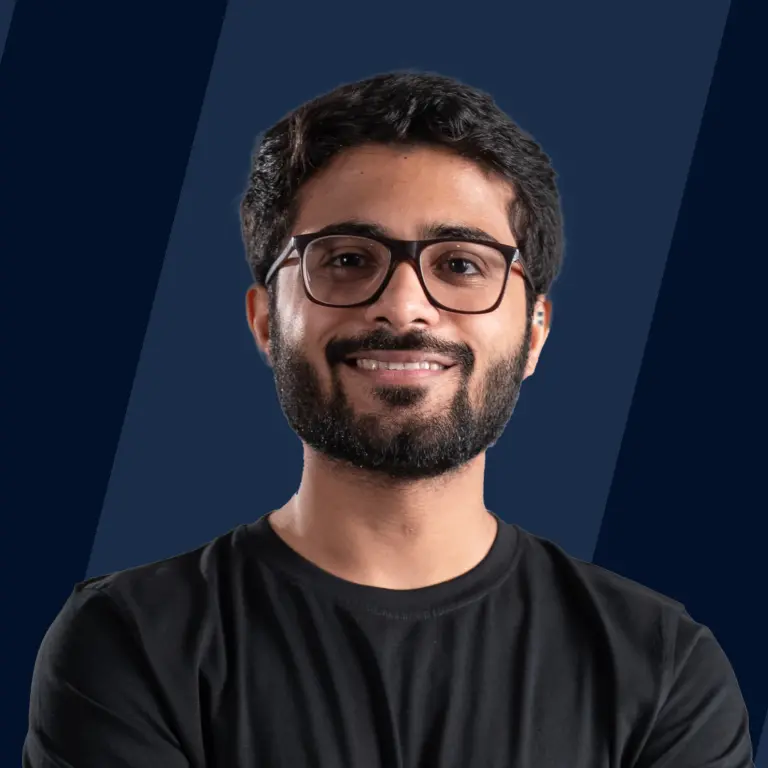
The revolutionary release of Java 8 in 2014 brought a massive upgrade to Programming in Java and a coordinated evolution of the JVM, Java libraries, and language. It brought several new features for productivity, security, improved performance, and ease of use, which changed Java programming.
These Java 8 Features include the forEach() method in the Iterable interface, Date/Time API, improvements in Collection API and Concurrency API, Java Stream API, default and static method in Interfaces, and many more.
Java 8 Features
Let us look at all these Java 8 features in Detail:
- Lambda Expressions
- Functional Interfaces
- Method Reference
- Stream API
- Comparable and Comparator
- Optional Class
- forEach
- Date/Time API
- Default Methods
- Java Parallel Array Sorting
- Miscellaneous
Lambda Expression
Lambda expressions can easily create anonymous classes of functional interfaces. In other words, it is a concise and straightforward way of representing one method interface or Functional Interface using an expression. It is convenient to use the collection library as it helps to iterate, filter, and extract data from collections like arrays, lists, etc.
Functional Interfaces
A functional interface in Java is an interface that contains only a single abstract (unimplemented) method. Along with a single abstract method, functional interfaces can have multiple default methods (interface methods with some default implementation, they can be overridden), static methods (methods in an interface having implementation, these can be used in implementing class but cannot be overridden as default methods), and methods of the object class. They can only exhibit one functionality.
Lambda Expressions can represent an instance of a functional interface to create an anonymous class and avoid bulky codes. Runnable, Callable, ActionListener, and Comparable are examples of functional interfaces in Java.
@FunctionalInterface annotation is optional, but it is a best practice to avoid accidentally adding abstract methods to Functional Interfaces.
Method References
Method references are a shorter, more readable way of lambda expression for only calling existing methods. They are compact, easy-to-read references that can be used to replace a single method of the lambda expression. Besides, in the method reference scope, resolution operator(::) separates the method name from the class name.
For Example:
can be rewritten with method references as below, without passing any parameters.
Stream API
Stream API is one of the essential Java 8 features. All the classes and interfaces of Streams API in Java are located in java.util.stream package. Streams API supports iteration of the elements of collections, where we can process them, perform operations on them, and store the output. Streams are functional, i.e. the changes made to elements do not modify the source.
Comparable and Comparator
In Java, Comparable is an interface for defining objects' natural ordering. It contains a single method, compareTo(), which enables objects to be compared. On the other hand, a comparator is an interface used to define custom comparison logic separate from the objects being compared. It contains a compare() method based on the custom logic provided for comparing two objects.
To learn more in detail about it visit: Comparable and Comparator in Java
Optional Class
Optional class is present in Java.util package. It is a public final class that avoids NullPointerExceptions in Java Applications. The optional class contains methods that provide easy and concise ways to check if some value is null and perform an action if it is null.
A few of the valuable methods of Optional class are described below:
- Optional.ofNullable() - if the given object is null, it returns an empty Optional; else, it returns a Non-empty Optional. As in our example, if the message is null, it will return an empty Optional.
- StringOptional.isPresent() - It returns true if Optional is Non-empty, it returns false. Thus, it prints Message is Empty.
- In the case of GoodMorning, it will be Non-Empty Optional. GoodMorning.ifPresent() - if Optional is Non-Empty it can perform some action to it. In our case, we simply print the Optional value, i.e. Good Morning .
- StringOptional.orElse() assigns a value only if the given Optional is Empty.
forEach
The forEach() method is defined as default in the Iterable Interface and is used to iterate through elements. Collection Interface extends Iterable Interface thus, classes implementing Collection Framework like Arrays, Lists, Stacks, etc. also extend Iterable Interface.
Syntax:
The forEach() method takes action to be performed on each element of a Collection as the parameter.
To learn more in details about forEach() visit: For-each Loop in Java
Date/Time API
Existing Date and Time APIs in Java were not thread-safe, and there was a lack of consistency as java.util and java.sql both packages define Date class, and it didn't have timezone support, so developers had to write an additional logic for timezone and thread-safety.
Java 8 introduced java.time package. The new Date and Time APIs are immutable and thus thread-safe, follow consistent domain models for the date, time, duration, and periods, and support Local and Zonal Date/Time APIs.
New Date and Time API have two important classes among them:
- Local - This class provides simple Date and Time operations in the Local Time Zone.
- Zoned - It contains Time zone-specific Date/Time and its operations.
Let us take an example to see various methods of Local and Zonal classes, like storing system date or time, performing some operations on them, etc.
Output:
Default Methods
From Java 8 onwards, interfaces can have declared methods along with abstract methods. Default Methods are declared using the default keyword. We do not require overriding these methods; they can be used directly. If we want a customized implementation, we can override the method's behaviour.
Java Parallel Array Sorting
The parallelSort() method is introduced in the Array class of java.util package. It uses the concept of multithreading to sort the array faster. It first divides the array into subarrays, sorted individually by multiple threads, and then merged.
Output:
Miscellaneous
Additionally, Java 8 introduced default methods in interfaces, allowing for backward compatibility while enabling interface evolution. These features collectively enhance code readability, maintainability, and expressiveness in Java development.
To learn more about them visit the below links:
Conclusion
- Java 8 brought many features and libraries that improved Java programming.
- Functional interfaces that have only one abstract method. Functional interfaces can be used to implement lambda expressions.
- Lambda expression provides a simple and compact implementation of an abstract functional interface method. It consists of three components, argument, arrow-token, and body.
- Method references concisely call existing methods instead of a lambda expression.
- Stream API introduced in Java 8 is helpful for iterations, filtration, performing operations, and storing the collection elements. Stream operations are of two types, Intermediate Stream operations and Terminal Operations.
- foreach() method supports iteration of elements of Collections like Array, ArrayList, Stack, Queue, etc.
- Java 8 also brought many new methods for Date and Time operations in Java. Two such vital classes are Local and Zoned.
- Static methods are also declared in Interfaces like default methods. But they cannot be overridden or changed.
- Optional class provides ways to avoid NullPointerExceptions. We can check if the value is null, provide a default value, and perform some action.
- Parallel array sorting uses the concept of multithreading and sorts the array faster.
FAQs
Q: What is Java 8 good for?
A: Java 8 introduced features like lambda expressions, streams, and functional interfaces, enhancing code readability and enabling better concurrency handling.
Q: What is the new feature of Java 8?
A: Java 8 introduced lambda expressions and the Stream API for functional-style programming.
Q: Is Java 8 free?
A: Yes, Java 8 is free to use.