Boolean Operators in Python
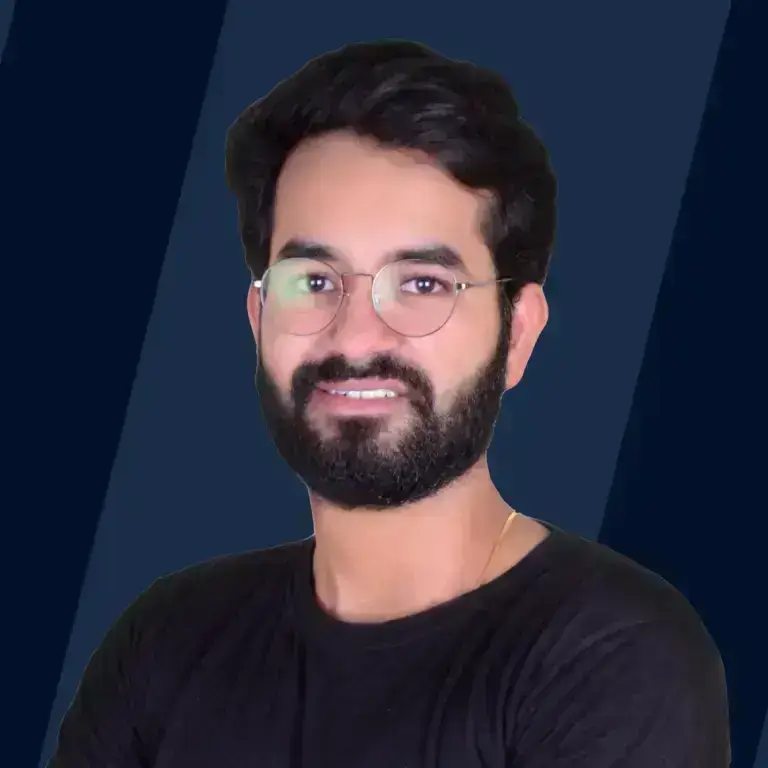
Abstract
Boolean Operators are those that result in the Boolean values of True and False. These include and, or and not. While and & or require 2 operands, not is a unary operator. Boolean operators are most commonly used in arithmetic computations and logical comparisons.
What are Boolean Operators in Python?
Since childhood, you might have come across True or False Quizzes. Since True or False represent 2 extremities that have a lot of significance in Mathematics and Logic, these values come under a different data type called Boolean. In programming, we use Boolean data type in comparisons and flow of control.
True or False are called Boolean values which are keywords in Python. Instructions that combine operators and values to perform mathematical or logical computations are called expressions.
Expressions that yield these Boolean values and are formed with operators called Boolean operators are called Boolean expressions. The operations that take place in the process are called Boolean operations.
Types of Boolean Operators in Python
There are two types of operators in Python that return boolean values, i.e., Logical operators and Comparison operators. Since both these operators have return types as boolean, they are also termed, Boolean operators.
1. Comparison operators
If you were to choose a stream between Science, Commerce and Humanities, you would weigh out their pros and cons and accordingly take a decision. When we want to decide between 2 or more options, we compare them based on their weights.
In programming, comparison operators are used to comparing values and evaluate down to a single Boolean value of either True or False.
Operator | Title | Description | Example | Output |
---|---|---|---|---|
== | Equal to | It results in a True if the 2 operands are equal, and a False if unequal. | print(1==1) print((10-5+2)==(7-9+1)) | True False |
!= | Not equal to | It results in a True if the 2 operands are unequal, and a False if equal. | print(3!=2) print((7-1*2)!=(8*2+4)) | True True |
< | Less than | It results in a True if the first operand is smaller than the second, else a False. | print(1<1) print((10-5+2)<(7-9+1)) | False False |
> | Greater than | It results in a True if the first operand is greater than the second, else a False. | print(1>1) print((10-5+2)>(7-9+1)) | False True |
<= | Less than or equal to | It results in a True if the first operand is lesser than or equal to the second, else a False. | print(3<=2) print((7-1*2)<=(8*2+4) | False True |
>= | Greater than or equal to | It results in a True if the first operand is greater than or equal to the second, else a False. | print(1>=1) print((10-5+2)>=(7-9+1)) | True False |
2. Logical Operators
The logical operators and, or and not are also referred to as Boolean operators. and and or require 2 operands and are thus called binary operators. On the other hand, not is a unary operator which works on one operand.
Operator | Description | Representation |
---|---|---|
and | True if both are True | x and y |
or | True if at least one is True | x or y |
not | True only if False | not x |
-
and It is a binary operator surrounded by 2 operands (variables, constants or expressions). It analyzes them and results in a value based on the following fact: ‘It results in True only if the operands on both sides of the AND are True.’
**Consider the statement: **
I will come to the party if mom is back from work and she permits me to leave.
The statement implies that I will go to the party only if both the conditions are satisfied.
Thus, let’s consider 2 example cases and the results based on the and operator.
Example:
Output:
-
or It is a binary operator surrounded by 2 operands (variables, constants or expressions). It analyzes them and results in a value based on the following fact: ‘It results in False only if the operands on both sides of the OR are False.’
Consider the statement:
I will rest if Mohan disconnects the call sooner or there is no extra class.
The statement implies that I will rest if both conditions are satisfied and not if none of them is.
Thus, let’s consider 2 example cases and the results based on the or operator.
Example:
Output:
-
not It is a unary operator that is used to negate the expression after succeeding the operator. According to this, every True expression becomes False, and vice versa.
Consider the statement:
Sunita will not go to school.
If the above statement is true, the statement ‘Sunita will go to school’ would be false.
Thus, not interchanges True and False values.
Example:
Output:
Truth Rables
So many different concepts might seem difficult to remember. Here is where truth tables jump in. Truth tables are used to summarize the results of using Logical operators in a tabular format. It consists of the operands and final statement in consideration.
Truth Table for and Operation:
X | Y | X and Y |
---|---|---|
False | False | False |
True | False | False |
False | True | False |
True | True | True |
Truth Table for or Operation:
X | Y | X or Y |
---|---|---|
False | False | False |
True | False | True |
False | True | True |
True | True | True |
Truth Table for not Operation:
X | not X |
---|---|
True | False |
False | True |
The above truth tables can also be combined as one based on the requirements and be compiled based on the expression in consideration.
Chaining Comparison Operators
Comparison operators can be chained to summarize the output of 2 or more operations. Thus, 1<3 and 3<4 can be written as 1<3<4 using the mechanism of chaining. This is in contrast to C language which evaluates 1<3<4 as (1<3)<4.
Example:
Output:
The types and operations in a chain can be mixed as long as they are comparable.
Example:
Output:
Chaining is useful while checking for ranges in real-world models. Say, to check if the grade is between 80% and 100%, you can chain comparison operators as given below:
Output:
Short-Circuit Chaining
Short-circuiting occurs when the execution of a boolean chain stops when the truth value of an expression has been determined. If chains use an implicit and, it means there will be some kind of short-circuiting caused too.
The statement print(3 < "2") gives an error while print(3 < 2 < "2") does not. This happens as even though Python cannot compare int and string, it compares the first part and gives the result accordingly. Short-circuit chains can also prevent exceptions like ZeroDivisionError.
Example
Output:
None as a Boolean Value
In Python, None is considered as a Boolean False. None can also be used as a default value in the case of Short-circuit chains.
Example:
Output:
Numbers as a Boolean Value
In Python, 0 is considered to be False. Every other number, whether positive or negative, is True. Even Inf and Nan are considered to be True.
Example:
Output:
Sequences as a Boolean Value
In Python, the len() value (Length) of objects is considered while evaluating their truthiness (whether True or False). Thus, an empty string, array or set will result in a Boolean False value.
Example:
Output:
Boolean operators can be used for various purposes. Some common usage scenarios for boolean operators can be stated as follows:
- Testing of conditions in if statements: Help evaluate whether you should get into the If block.
- Can be used as conjunctions to exclude or include Keywords in Search: and helps Narrow down searches, while or broadens the search results.
Conclusion
The Boolean operators in Python are widely used and have numerous applications in functions and conditional statements. Logical operators like and, or, not and comparison operators like ==, !=, >,<, >=, <= are used to compare values and result in Boolean Outputs of True and False. Truth tables are used to summarize the outputs of these operations. Comparison operators can also be chained for ease of coding but might lead to short-circuiting.