Literals in Python
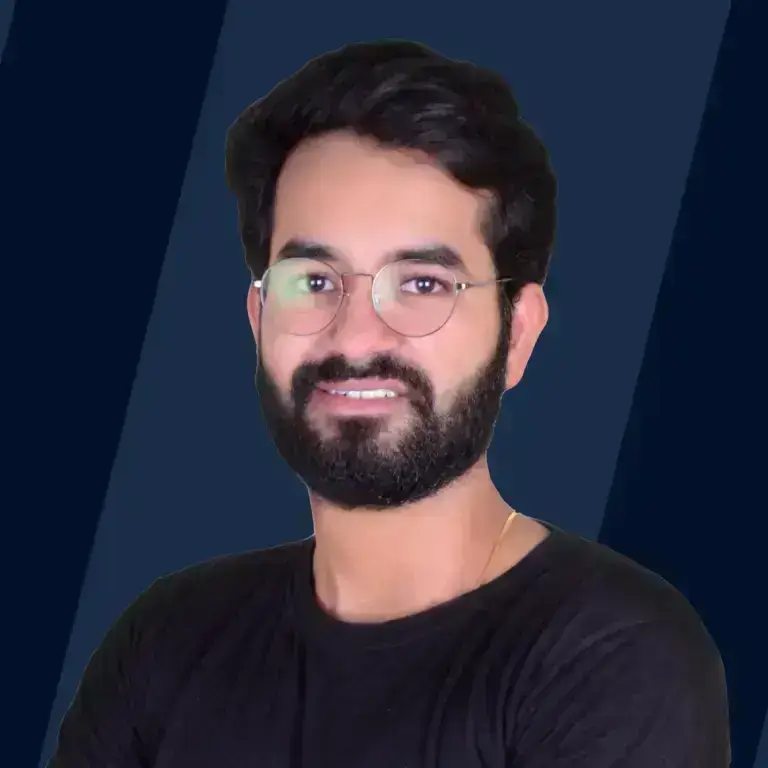
Overview
Literals in Python is defined as the raw data assigned to variables or constants while programming. We mainly have five types of literals which includes string literals, numeric literals, boolean literals, literal collections and a special literal None.
Introduction to Literals in Python
Be it a friend, family member or a cousin you’re really close to, we all need a constant in life. Well, the same applies to programming languages like Python!
Jokes apart, even if we speak mathematically, a constant can be defined as-
“A constant can be defined as an entity that has a fixed value or an entity whose value does not vary.”
Well, we luckily have the concept of constants in Python as well! They are known as literals in Python.
Python literals are quantities/ notations whose value does not change during the execution of a program.
In this article, we will be deep-diving into what are literals in Python and the types of literal in Python.
Literals in Python are nothing but a succinct way of representing the data types. In simpler words, it is the way to represent a fixed value in our source code. They can either be numbers, text, boolean or any other form of data.
Types of Literals in Python
Python literals are of several types, and their usage is also pretty varied. So let’s check them out one by one.
There are five types of literal in Python, which are as follows-
- String Literals
- Numeric Literals
- Boolean Literals
- Literal Collections
- Special Literals
And these five types again have certain subtypes, which we will discuss in-depth and see their implementation in Python.
1. String Literals in Python
Let’s discuss string literals in Python!
Creating a string literal in Python is really easy- enclose the text or the group of characters in single, double or triple quotes. Using triple quotes also allows us to write multi-line strings.
Hence, we can say that there are two types of string literals in Python-
Single-line String
String literals that are enclosed within single quotes (‘’) are known as single-line strings.
Now, let’s look at an example to understand how they work-
We have successfully created a string using single quotes and double quotes in the above code snippet. The output of it is-
Multi-line String
A collection of characters or a string that goes on for multiple lines is a multi-line string.
These kinds of strings are again implemented in two types-
a) Adding backslash at the end of every line-
We can enable multi-line strings in Python by adding a backslash at the end of every line.
Let’s see how it's done-
The output of this will be-
b) Using triple quotes-
Triple quotes at the beginning and end of the string literally will allow us to create a multi-line string in Python easily!
Let’s see the code for it-
The output will be-
2. Numeric Literals in Python
Numerical literals in Python are those literals that contain digits only and are immutable.
They are of four types-
Integer
The numerical literals that are zero, positive or negative natural numbers and contain no decimal points are integers.
The different types of integers are-
- Decimal- It contains digits from 0 to 9. The base for decimal values is 10.
- Binary- It contains only two digits- 0 and 1. The base for binary values is 2 and prefixed with “0b”.
- Octal- It contains the digits from 0 to 7. The base for octal values is 8. In Python, such values are prefixed with “0o”.
- Hexadecimal- It contains digits from 0 to 9 and alphabets from A to F.
Now, let’s see some examples-
Check out the output for this-
Float
The floating-point literals are also known as real literals. Unlike integers, these contain decimal points.
Float literals are primarily of two types-
A. Fractional- Fractional literals contain both whole numbers and decimal points.
An example of fractional literals will look like this-
Example
Output
B. Exponential- Exponential literals in Python are represented in the powers of 10. The power of 10 is represented by e or E. An exponential literal has two parts- the mantissa and the exponent.
Note:
- Mantissa-The digits before the symbol E in an exponential literal is known as the mantissa. In computing, it denotes the significant digits of the floating-point numbers.
- Exponent- The digits after the symbol E in an exponential literal are the exponent. It denotes where the decimal point should be placed.
Let’s look at an example-
Example
Output
Complex
Complex literals are represented by A+Bj. Over here, A is the real part. And the entire B part, along with j, is the imaginary or complex part. j here represents the square root of -1, which is nothing but the iota or i we use in Mathematics.
The output of the code snippet will be-
Long
Long literals were nothing but integers with unlimited length. From Python 2.2 and onwards, the integers that used to overflow were automatically converted into long ints. Since Python 3.0, the long literal has been dropped. What was the long data type in Python 2 is now the standard int type in Python 3.
Long literals used to be represented with a suffix- l or L. The usage of L was strongly recommended as l looked a lot like the digit 1.
Check out the following example to see how it was denoted-
Example
Note– The code snippet was executed using Python 1.8. Output
3. Boolean Literals in Python
Boolean literals in Python are pretty straight-forward and have only two values-
- True- True represents the value 1.
- False-False represents the value 0.
Let’s see an example-
The expected output is-
We can see that we used boolean literals for comparison and based on the conditions, we received the outputs True and False, respectively.
4. Special Literals in Python
Python literals have one special literal known as None. This literal in Python is used to signify that a particular field is not created.
Python will print None as output when we print the variable with no value assigned to it. None is also used for end of lists in Python.
Example
Output
5. Literal Collections in Python
If we wish to work with more than one value, then we can go for literal collections in Python. Literal collections in Python are of four types-
List Literals
Lists are a collection of data declared using the square brackets([]), and commas separate the elements of the list (,). This data can be of different types. Another important thing to know about lists is that they are mutable.
Now let’s see an implementation-
Output
Tuple Literals
The literals that are declared using round brackets and can hold any data type are tuples. Commas separate the elements of tuples. However, unlike lists, tuples are immutable.
Let’s check out a code snippet on tuples-
Output
Dictionary Literals
Dictionary is a collection of data that stores value in a key-value format. These are enclosed in curly brackets and separated by commas. Dictionaries are mutable and can also contain different types of data.
Check out the below code snippet that shows how a dictionary works-
Output
Set Literals
Set literals are a collection of unordered data that cannot be modified. It is enclosed within curly brackets and separated by commas.
Let’s see the code for this-
Output
Conclusion
Now that we have reached the end of the article, you can positively say you know what literals in Python are!
Along with that, you also got to learn about-
- The different types of literals.
- How to use Python literals.
- In-depth knowledge about the nature of Python literals.
So wait no more and get coding!