Python sorted() Function
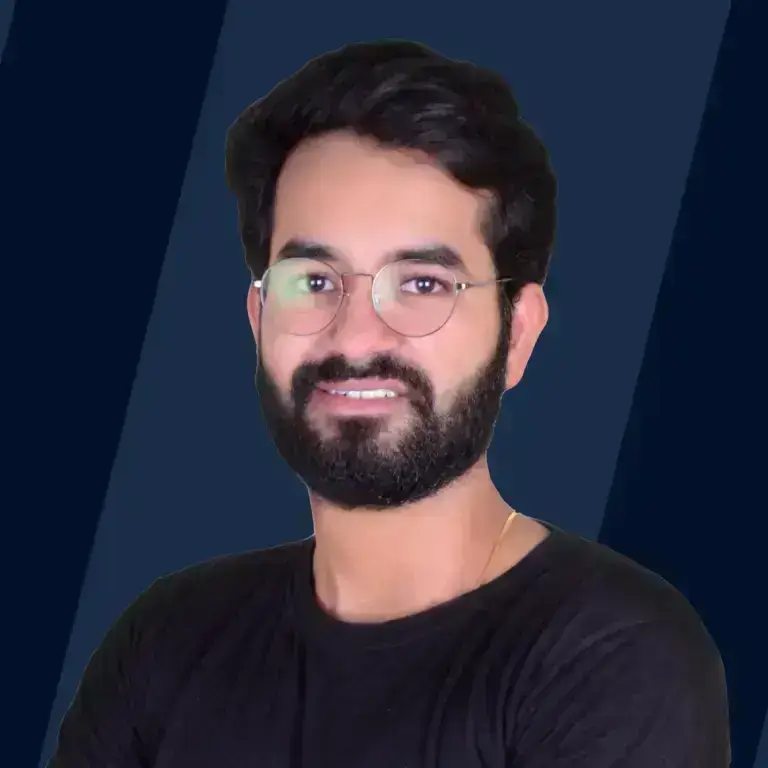
The sorted() function in Python is a library function that is used to sort an iterable object and returns an iterable containing all the elements of the passed iterable object in a sorted manner.
The iterable object is simply the object which can be iterated on, whether it be a list, dictionary, tuple or string, etc.
Syntax of sorted() function in Python
The syntax of Python sorted() Function is as follows:
Parameters of sorted() function in Python
Below are the parameters of the sorted function:
- iterable_object: It is the iterable object which needs to be sorted. It can be a sequence like string and list or any collection like set and dictionary or any other iterator object.
- reverse (Optional): It specifies the sorting order, i.e., if the reverse is True, then descending, otherwise ascending.
- key (Optional) It is the function that provides the key index by which the sort comparison takes place.
For Example, if the array is [[2, 1], [1, 3]] and the key provided by this function is 0, then the sorted list will look like [[1, 3], [2, 1]] as sort comparison is according to the 0th index of all sublists.
Return Value of sorted() function in Python
Return Type: list
It returns a sorted list, according to the iterable passed, a list, tuple, dictionary, etc.
Example of sorted() function in Python
Output:
Explanation:
The myList is passed to the Python sorted() Function, which returns the sorted list, and the print function prints the return value, which is nothing but a sorted and duplicate version of the provided list.
How to Use sorted() Function in Python?
The sorted() function in Python is a built-in utility that allows you to sort the elements of an iterable (such as a list, tuple, or string) in a specific order (either ascending or descending) and returns a new sorted list from the items in the iterable.
Example
Output:
More Examples
Example 1: Sorting a String and Tuple
Output:
Explanation:
The characters of the string are being sorted according to ASCII value and the tuple of the integer is being sorted according to the integral values of elements.
Example 2: Using the reverse parameter
Output:
Explanation:
In all 3 cases: list, string, and tuple values are sorted and then those sorted values are reversed, and then reversed sorted list is returned.
Example 3: Using the key parameter
In this example we are going to utilize the key of the sorted function.
Output:
Explanation:
- The sorted() function is getting called with the key function which returns the key value by which the list is to be sorted.
- In 1st case, list is sorted according to 1st index of the sublists.
- In 2st case, the tuple is sorted according to 0th index of the subtuples.
- In 3rd case, the list is sorted according to 0th index of the sublists.
Example 4: Sorting a dictionary using the sorted function.
Here we are going to sort a dictionary with the sorted function let's see the consequences.
Output:
Explanation:
This dictionary is sorted according to the key and a list which contains all the sorted keys of the dictionary is being returned by the sorted function.
Example 5: Python Sorted() with lambda
Output:
Explanation: The sorted() function then sorts the elements based on their lengths, resulting in the list ['red', 'blue', 'green', 'yellow'], where elements are ordered from the shortest to the longest.
Example 6: Sorted() in Python with len()
Output:
Explanation: Here, the sorted() function has the built-in len function as the key. This instructs sorted() to arrange the elements in words based on their length, resulting in a list where 'date' comes first as the shortest word, followed by 'apple', 'banana', and 'cherry' in ascending order of length.
Example 7: Sorting a list in ascending order with sorted()
Output:
Explanation: This example uses the sorted() function to sort a list of numbers. By default, sorted() sorts the elements in ascending order, resulting in [1, 1, 2, 3, 4, 5, 9].
Example 8: Sorting a List of Custom Objects
Output:
Explanation: In this example, we have a Fruit class with name and quantity attributes. We create a list of Fruit objects and then sort it using the sorted() function with a lambda function as the key. The lambda function specifies that the sorting should be based on the quantity attribute of each Fruit object. The final list is sorted in ascending order based on quantity.
Conclusion
-
The sorted() in python is used to store a sorted copy of an iterable object in the form of iterable.
-
It takes 3 parameters: iterable_list, reverse, and key.
-
Finally, the iterable returned can be iterated over, and all the sorted values can be easily accessed in that list.
-
This is a very useful function because it works well with immutable data types also. The reason is, that it creates a new iterable object for sorted data.