Sort Dictionary by Value in Python
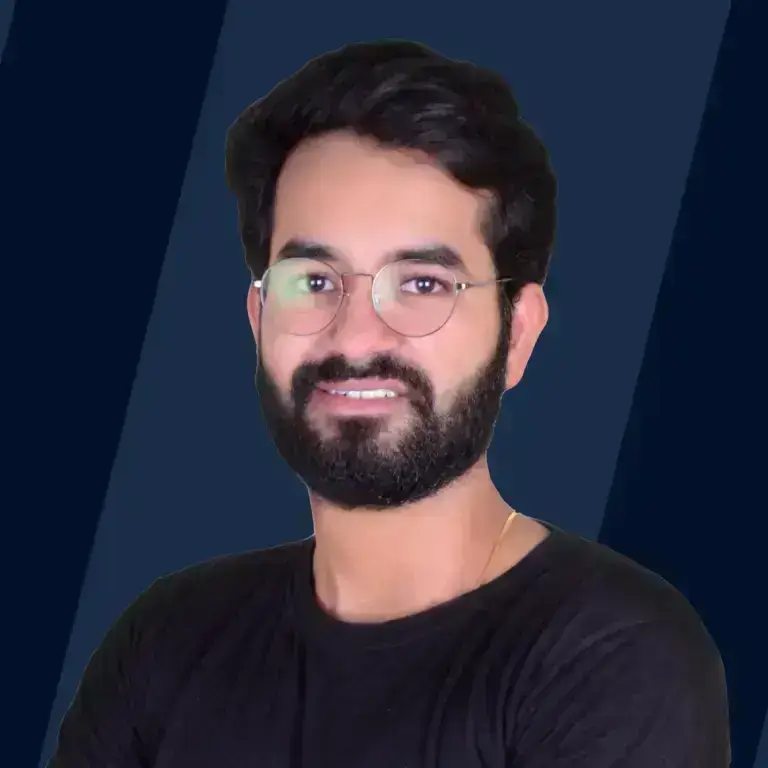
A dictionary is a fundamental data structure in Python that stores data in key-value pairs. Initially, a dictionary is an unordered set of data, but we can sort it with a key or value. Each key within a dictionary is unique, and it maps to a specific value. Utilizing a dictionary allows quick access to values using their associated keys with an efficiency of O(1).In this article, we will cover the different ways we can sort dictionary by value in Python.
For instance, when managing sales data for a shop, instead of using separate lists for item names and quantities sold, a dictionary can be employed. This optimizes the process, particularly in Python. In this case, items serve as keys, and their corresponding quantities sold are the values, such as:
By accessing the dictionary using item names, like shopItems['Bread'], the quantity sold can be retrieved. To sort the dictionary by its values, Python provides mechanisms for sorting based on either keys or values.
This approach condenses the concept of leveraging dictionaries for efficient data storage and retrieval while introducing the concept of sorting by dictionary values in Python.
Need to Sort the Python Dictionary by Value
Sorting a Python dictionary by value is valuable for several reasons.
- Data Accessibility and Efficiency: When handling sizable datasets, a sorted dictionary enhances data retrieval efficiency. Sorted values enable quicker access to relevant information, promoting streamlined data analysis and processing.
- Structured Output: Sorting facilitates structured output presentation, rendering information in an organized manner. This aids readability and enhances the user experience, especially when dealing with large amounts of data.
- Optimized Searches: Sorting empowers optimized search operations. It allows for easier identification of specific values or patterns within the data, leading to faster and more accurate results.
- Enhanced Data Analysis: For tasks like identifying top performers, trends, or anomalies, a sorted dictionary provides insights by placing relevant information at the forefront.
Methods to sort dictionary by value in Python
Here, we have different types of methods to sort dictionary by value in python:
- Firstly Convert the data in dictionary to a list and then perform sorting over the list
- Using Bubble Sort
- Using sorted() Method
- Using itemgetter() Method
- Using dict.items() and sorted() Functions
- By performing operations directly on the dictionary and
- Using a for Loop
- Using the sorted() Function
- Using a Lambda Function
- Using dictionary.items() Method
1. Convert the data in dictionary to a list
Let's begin by converting the data within the dictionary into a list format. Once we have the data in list form, we can proceed to sort the Python dictionary using various methods. Example:
Output:
By using the list() function, we convert the dictionary into a list of tuples where each tuple contains a key-value pair from the original dictionary. Once we have this list, we can perform sorting operations. Essentially, converting the dictionary into a list allows us to work with its elements in a different format, providing flexibility in data handling and analysis.
Using Bubble Sort
Here we can implement the Bubble Sort algorithm to sort the list of tuples based on the second element (quantity) of each tuple. Once you have the sorted list of tuples, you can convert it back into a dictionary
Example:
Output:
In this code, the dict() function is used to convert the sorted list of tuples back into a dictionary. The resulting dictionary sorted_fruit_basket contains the fruits sorted based on their quantities. Finally, the sorted dictionary is printed.
Using sorted() Method
We can achieve the same result using the sorted() method:
Example:
Output:
In this code, the sorted() method is used to sort the dictionary by its values (quantities). The key parameter is set to a lambda function that specifies the sorting criteria based on the second element (quantity) of each tuple. The sorted list of tuples is then converted back into a dictionary, and the sorted dictionary is printed.
Using itemgetter() Method
We can achieve the same result using the itemgetter() method from the operator module. Example:
Output:
In this code, the itemgetter() method from the operator module is used to specify the sorting key based on the second element (quantity) of each tuple. The sorted() function then sorts the dictionary items based on this key. Finally, the sorted list of tuples is converted back into a dictionary, and the sorted dictionary is printed.
Using dict.items() and sorted() Functions
We can achieve the same result using the dict.items() method and the sorted() function. Example:
Output:
In this code, the dict.items() method is used to obtain a view of the dictionary items as a sequence of tuples. The sorted() function then sorts these tuples based on the second element (quantity) using a lambda function as the key. Finally, the sorted list of tuples is converted back into a dictionary, and the sorted dictionary is printed.
2. By performing operations directly on the dictionary
Sorting a Python dictionary can be accomplished directly without the need to convert its items to a list. Here are the methods to achieve this:
Sort a Dictionary in Python Using a For loop
A for loop can easily sort a dictionary in Python by just sorting the values in the dictionary and then creating a new dictionary based on the sorted order of values present in the dictionary.
If we talk about the time and space complexity of the above approach, then the time complexity would be O(n^2), whereas the space complexity would be O(n).
This is not an in-place sort as we are creating a new dictionary to add the sorted values. Let's have a look at the example given below:
Example:
Output:
In the above example, we can see that first we have created a list of all the values in our dictionary and then we start traversing through all the values along with traversing the keys of the dictionary every time. We will perform matching of the keys and values, and finally, we will insert them into a new dictionary in the sorted form. At last, we have sort dictionary by value in Python.
Sort a Dictionary in Python Using Sorted() Function
Python is a function-enriched language. we can easily sort dictionary by value in Python. It has an inbuilt function sorted that can be used to sort a dictionary by using a parameter key. The key parameter in the sorted function is used to tell the function the deciding value according to which dictionary needs to be sorted.
To sort the dictionary using the sorted function, we will pass the value dict_name.get as the value for the parameter key. This will tell the sorted function that we need to sort the keys according to the values we get from the dictionary.
The primary logic behind the approach is to create a list of keys that are sorted according to their values and then form a sorted dictionary.
Code:
Output:
Sort a Dictionary in Python Using lambda Function
To sort a function using the lambda function, we will also need to use the sorted function. A lambda function is used as a value for the key parameter in the sorted function. As we discussed earlier, that key parameter in the sorted function defines the value through which sorting takes place. Here the lambda function works on getting that value.
We also use the .items() method in dictionary, which is used to create a list of tuples that represent a dictionary.
The primary logic behind this approach is to create a list of tuples and then sort them using sorted and lambda functions by using each tuple's second value as the key and then convert the sorted list of tuples into a new dictionary.
Code:
Output:
Using dictionary.items() Method
To sort a function using the operator module and itemgetter() function, we will also need to use the sorted function. The operator module and itemgetter() functions are used to define a value for the key parameter in the sorted function. As we discussed earlier, that key parameter in the sorted function defines the value through which sorting takes place. Here the lambda function works on getting that value.
We also use the .items() method in Dictionary, which is used to create a list of tuples that represent a dictionary. We also need to import the Operator Module before using it in the sorted function.
The Main Logic Behind this approach is to create a list of tuples and then sort them using the sorted, operator module, and itemgetter() Function by using each tuple's second value as the key and then converting the sorted list of tuples into a new dictionary.
Note: This method is very similar to sorting a dictionary in Python using the lambda function. The only difference between them is that instead of using lambda to get the second value for each tuple in the list of tuples, we use the Itemgetter function to access the 1st index element, i.e., the second element for each tuple to sort dictionary by value in Python
Code:
Output:
Sort Python Dictionaries by Value Example
Sort the Python Dictionary by Value in Ascending or Descending Order
We use the sorted() function to sort the dictionary items based on their values (x[1]). For ascending order, we use the default behavior of sorted(). For descending order, we set the reverse parameter of sorted() to True. The sorted items are then converted back into dictionaries using the dict() constructor. Finally, we print the sorted dictionaries.
Example:
Output:
In above example we have python sort dictionary by value in ascending and descending order.
Conclusion
Let's conclude our topic, sort dictionary by value in Python.
- A Python dictionary stores key-value pair mappings, where the key must be unique.
- Bubble Sort: Basic but slow sorting method involving repeated comparisons and swaps.
- The sorted() method takes 3 parameters - object, key and reverse. sorted() sorts the objects in ascending order by default. To sort the object in reverse order, we set the reverse parameter in sorted() method to True.
- A dictionary can be sorted using predefined methods like dict.values() and dict.items() as well as by custom sort functions.
- The operator module and itemgetter() functions are used to define a value for the key parameter in the sorted function.