Sort Array in Python
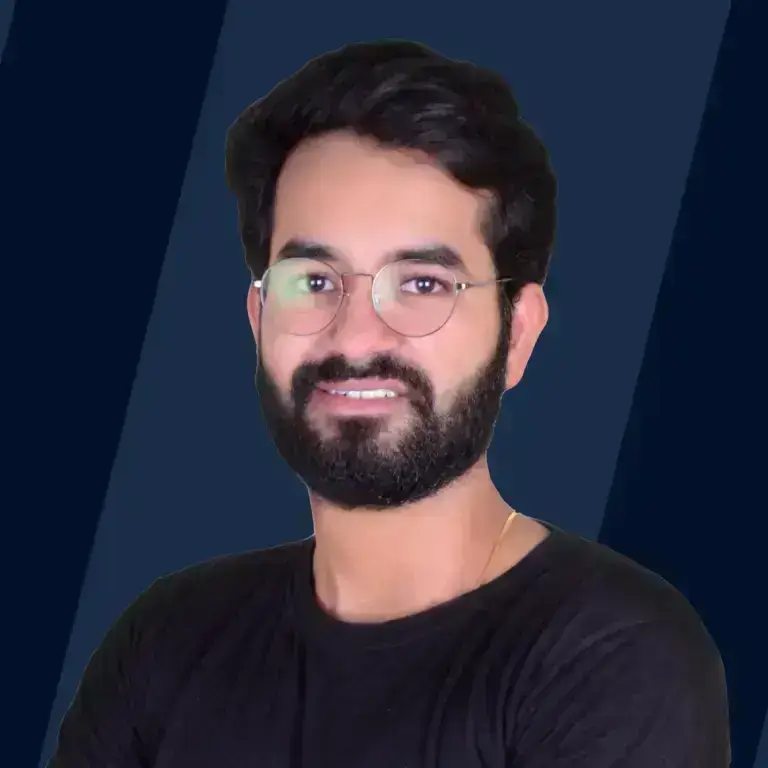
Sorting arrays is a fundamental operation that enhances data analysis and organization, whether you're arranging numbers, strings, or even complex objects. Python provides built-in methods like the sort() function for in-place sorting and the sorted() function for creating a new sorted list from an iterable.
Moreover, for specialized sorting needs or educational purposes, you can implement custom sorting algorithms such as bubble sort, insertion sort, or selection sort. To grasp the foundational concepts of arrays that underpin these sorting techniques, consider exploring Array in Python as a prerequisite.
Sort array in Python Using sort() Function
To sort an array in Python, the sort() method is a direct and efficient approach.
Syntax:
Parameters:
- key: Optional. A function to execute to decide the order. Default is None.
- reverse: Optional. A Boolean value. False will sort ascending, True will sort descending. Default is False.
Return:
The sort() method doesn't return any value. Instead, it modifies the original array in-place.
Examples:
-
basic example of Python sort() method:
Sort an array of integers.
Output: [3, 5, 6, 8] Explanation: The integer elements in the array are sorted into ascending order, showcasing a simple application of the sort() method to sort array in python.
-
Sort the array alphabetically Sorting an array of strings alphabetically is another common task. This example demonstrates how to sort an array of strings alphabetically, which is a straightforward way to sort array in python by character order.
Output: ['Apple', 'Banana', 'Cherry'] Explanation: The strings in the array are sorted alphabetically.
-
Sort a boolean array: Boolean arrays can also be sorted in Python, where False values precede True values. This example illustrates how to sort a boolean array, which is a unique case of how to sort array in python based on boolean values.
Output: [False, True, True] Explanation: The boolean array is sorted with False coming before True.
-
sort() method on a 2-D array: Sorting a 2-D array based on a specific element, such as the first element of each sub-array, requires a slightly different approach using the key parameter. This example shows how to sort a 2-D array in Python, focusing on a specified element within each sub-array to sort array in python effectively.
Output: [[1, 4], [2, 5], [3, 6]] Explanation: The 2-D array is sorted based on the first element of each sub-array, resulting in ascending order."
sort array in Python Using sorted() Function
The sorted() function in Python offers a convenient way to sort arrays and is particularly useful because it returns a new sorted list from the iterable passed as an argument, without modifying the original array.
Syntax:
Parameters:
- iterable: The sequence to sort (list, tuple, string, etc.).
- key: Optional value. It is a function that acts as a key for the sort comparison.
- reverse: Optional. Set to True for descending order, and False (default) for ascending.
Return:
A new sorted list from the provided iterable.
Example:
Sorting an Array of Numbers: Suppose you have an array of numbers and you want to sort them in ascending order.
**Output: **[4, 8, 12, 20] Explanation: The sorted() function returns a new list with the elements in arr sorted in ascending order, leaving the original array unchanged.
For more intricate details and examples on using the sorted() function in Python, you can visit here.
Sort array in Python Using a sorting algorithm
Sorting an array in Python can also be achieved by implementing a custom sorting algorithm. This method is helpful in understanding the mechanics of sorting and for cases where specific sorting behavior is required that isn't directly supported by built-in functions.
Bubble Sort Example:
It is one of the simplest sorting algorithms; in this algorithm, the array is sorted by comparing adjacent elements; the elements are swapped if they are in the wrong order, repeating the process until the array is sorted.
Code:
Output:
Explanation: The function bubbleSort iterates over the array, comparing each pair of adjacent items and swapping them if they are in the wrong order. The pass through the array is repeated until no swaps are needed, indicating the array is sorted.
Conclusion
- Sorting arrays in Python enhances data management, making the sort() and sorted() functions indispensable tools for developers.
- Custom sorting algorithms like bubble sort, insertion sort, and selection sort offer deeper insights into how sorting operations are conducted beneath the surface.
- Understanding arrays, as discussed in Array in Python, is crucial for effectively implementing and utilizing sort functionalities in Python.