Sort a String in Python
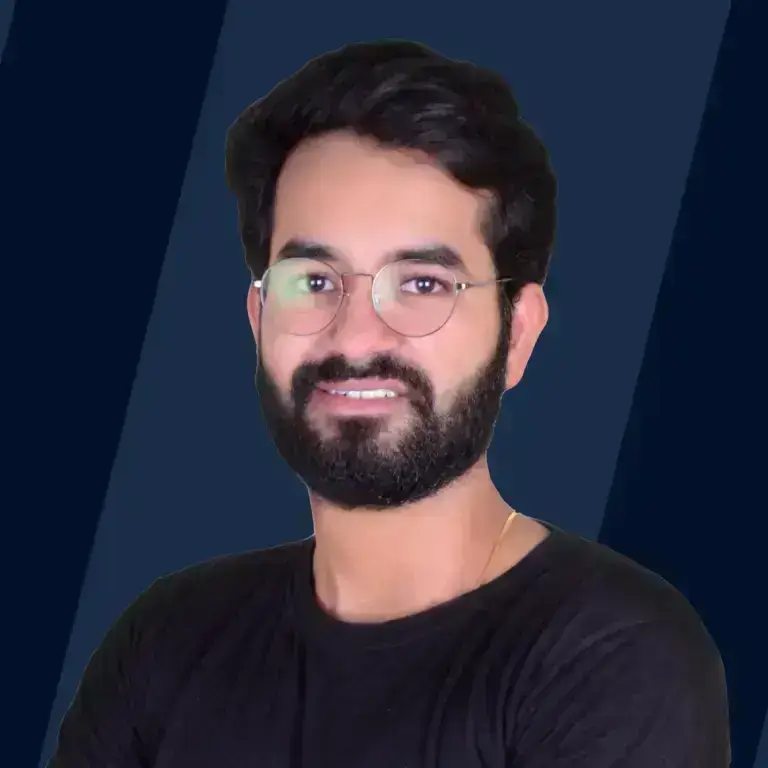
Overview
Every programmer might have faced the need of sorting data at some point in time. In this article, we will discuss about how to sort a string in Python. A string can be sorted by arranging its characters in a specific order, i.e. ascending or descending, based on their ASCII values. After sorting a string, we get an output that is arranged in a certain order.
Introduction
Sorting a string in Python is the process of placing a group of characters in a particular order, that can be ascending or descending based on their ASCII values.
Note: ASCII is a 7-bit character set containing 128 characters. It contains the numbers from 0-9, the upper and lower case English letters from A to Z, and some special characters, which are used by modern computers.
The result we get after sorting a string is in a definite order.
Above given is an example of sorting a string. When we sort the string, they are arranged in an alphabetical order, which our python code decides on the basis of their ASCII values. The character which comes first in alphabetical order has a smaller ASCII value than the character that comes later and vice versa.
After having learned about what is sorting in Python, let's see the various ways to sort a string in Python.
Using Python Inbuilt Sorted() and Join() Method
The sorted() function in Python returns a "sorted list" of the given iterable (list,tuple,set,etc.) object. We can also specify ascending or descending order in our function.
The join() method takes all items in an iterable (list,tuple,set,etc.) and joins them into one string. It joins the string on the basis of the separator provided. Separator means the value which will be used to join the string, suppose a space(" "), or a dash("-"), etc. Based on the separator provided, our join method joins the string with it.
Hence, we can use the combination of sorted() and join() method to sort our string. The sorted() will return a sorted list, and the join() will join the sorted list into a string.
Code:
Output:
Explanation:
- We pass our string to sorted() and it returns a sorted list of characters. We store the result in our variable ls.
- We finally pass our list to join() method and join it using the separator ''. Finally, we return our answer.
Time Complexity:
- Since we are using sorted() function, which in the worst case takes to sort, the time complexity becomes .
Space Complexity:
- In the above code we are using a list to store the sorted list result, so the space complexity is O(N), where N is the length of the list. However, in the best case we may avoid assigning it to a list and directly pass the sorted result to join. In that case our Space Complexity will be O(1).
Using Sorted() + Reduce() + lambda
The reduce(fun,seq) function is used to apply a particular function passed in its argument to all of the list elements mentioned in the sequence passed to it. This function is defined in functools module.
Note: It works only for python 2.
In Python, anonymous functions(functions without name) are defined using the lambda keyword. Lambda functions can have any number of arguments but only one expression. The expression is evaluated and returned.
Now, let us see how can we use sorted() + reduce() + lambda to sort our string in Python.
Code:
Output:
Explanation:
- First we sort our string using sorted and store its result in 's'.
- lambda x, y: x + y concatenates the list. The reduce method applies this lambda function to all the elements of the list.
- Here we join the resultant sorted list of characters using the lambda function and the reduce() function.
Using While loop in Python
We can also use the regular while loop method to sort our string in Python. However, it is not at all a suggested method. You can just understand this for the sake of knowledge.
Code:
Output:
Explanation:
- We pass our list of string to our sort_string method. Here, we loop over our list and append the minimum string according to the ASCII value of the first and leading characters of the string.
- Once we append that string to our list, we remove that string from our original string provided.
- Finally we return our list once it exits the while loop.
Time Complexity:
- The while loop would iterate over all the list elements hence take a complexity of , for list of size N. The min and max operations almost takes constant time for a list of constant size.
Space Complexity:
- The space complexity for the above code is . Because we are using a list of length 'n' to store our result and for computation.
Using For loop in Python(Bubble Sort)
We can use bubble sort to sort our string in Python. Let us see the code for how it works.
Code:
Output:
Explanation:
- First, i iterates through the length of the string, and j iterates through (length - i), because with every pass, the largest string will find it's position.
- Whenever we encounter a larger character in a string than the string after it, we simply swap them.
- Finally we return our sorted string.
Time Complexity:
- Since bubble sort uses to sort any iterable, it's worst case time complexity will be . The best and average case for bubble sort is also .
Space Complexity:
- Since bubble sort uses constant space the Space Complexity would be . Also, we are not using any other data structure to perform our operation.
Conclusion
Here we saw how to sort a string in Python.
- Sorting a string in python means arranging string into a particular order.
- We can sort a string by using sorted() to sort and join() to join the list returned by sorted().
- We can also use bubble sort by iterating through the string and comparing them while sorting on the way.
- sorted, lambda and reduce can be used to sort a string in Python by first applying sort and then lambda to join the string, finally using reduce to apply lambda over all the strings.
- While loop can also sort a string by appending the minimum string to a list at a time and removing that from the original string. However, it is not a recommended method.