Sort Dictionary by Key in Python
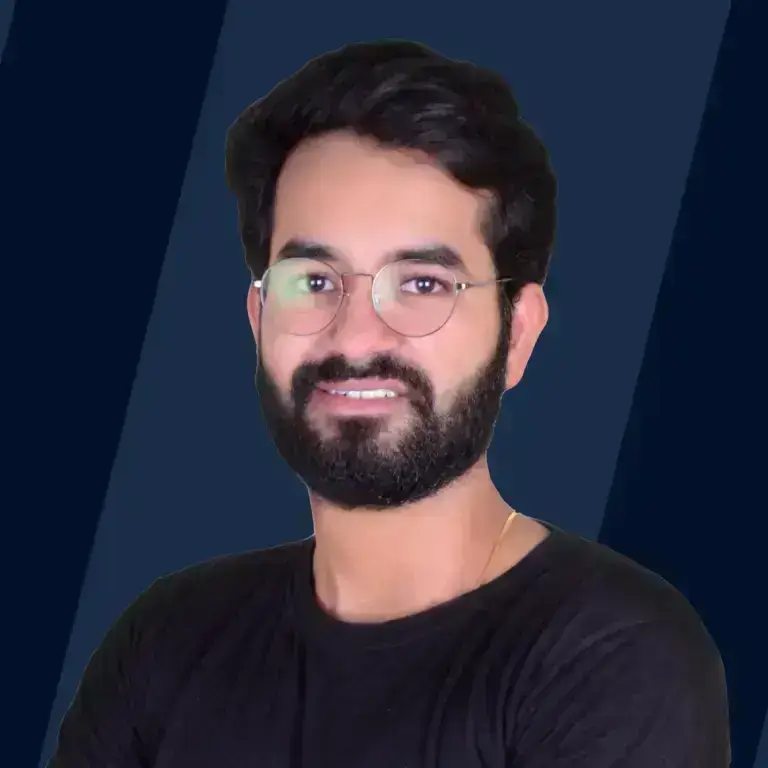
Overview
A Python dictionary is a data structure mapped as key-value pairs. Initially, a dictionary is an unordered data set, but we can sort it using either key or value. This article will cover how we can sort a dictionary in Python. We will use pre-defined functions in Python like dict.keys() and dict.items() as well as custom key functions to sort a Python dictionary.
Introduction to Sort Dictionary by Key in Python
A dictionary in Python is used to store key-value mappings of data. Multiple key-value pairs (key: value) are separated by commas and stored within curly braces. You might be wondering what the need is for a dictionary when we already have so many data structures like list, set, and tuples already!
Let us imagine we need to create a report card with all the subjects and the marks we scored. One way is to have two lists - one for subjects and one for marks. The i-th index of marks[] list will store your score in the subject at the i-th index of the subjects[] list. That does the task, right? But is there a more optimized way of accessing the subject-wise marks?
You guessed it, Dictionary! We can create a dictionary with subjects as keys and corresponding marks as values. This is what a Python dictionary looks like:
We have our report card ready. We can now access our subject marks simply by passing the subject name (key) as a subscript to our dictionary.
Output:
Now let's suppose we want to sort our report card in the alphabetical order of subject names for better clarity. Or maybe we want to sort it with our highest marks first. This is where sorting comes into the picture. In Python, we can sort a dictionary both by key (in this case, subject name) and by value (marks scored).
How to Sort a Dictionary?
A dictionary can be sorted in many ways, as listed below. We will cover each of these in detail in the subsequent sections.
- Sorting by keys
- Sorting by values
- Reversing the sorted order
- Custom sorting algorithms
Sorting by Keys
We can sort a dictionary by keys using various pre-defined and custom functions. Let us use our Report Card problem for all our further examples.
Before diving into the various techniques of sorting a dictionary in Python, let us first learn about the sorted() method.
As you might have guessed, sorted() is used to sort iterable objects using a key. It sorts the items in an iterable (list, tuple, dictionary) and creates a new iterable object sorted.
SYNTAX:
The sorted() method takes three parameters:
- Object: Iterable object that needs to be sorted
- Key (optional): Custom function according to which we want to sort our object.
- Reverse (optional): Boolean value to specify if we need to sort in reverse / descending order. It is False by default.
EXAMPLE:
In the above example, report.items() is the object that must be sorted. We use a Lambda function x[1] as the key to sort the object and set reverse to True so that the object is sorted in reverse order. Do not worry about these terms; we will cover them in detail in the upcoming sections.
We can sort a dictionary by keys using the following methods:
- Sort using dict.keys()
- Sort using dict.items()
- Sort using custom key functions
1. Sort using dict.keys()
The dict.keys() method is used to return the keys in a dictionary called dict.
Remember that our dictionary storing subject-marks pairs is called report. We can use the sorted() function and pass report.keys() as the object to be sorted.
SYNTAX:
EXAMPLE:
To print the dictionary displaying the subject name and marks, we can iterate over the sorted list of keys and access the corresponding marks.
Code:
Output:
2. Sort using dict.items()
We have another method called dict.items(), which returns the key-value pairs of a dictionary as a list of tuples. This is different from dict.keys(), which only returns the keys as a list.
SYNTAX:
We can pass report.items() as the object in the sorted() function as:
It will sort the list based on the 0-th index of the tuple (here, subject name, or the key).
If we print the above, we get a sorted list storing the subject name and corresponding marks as tuples:
EXAMPLE:
To print the dictionary displaying the subject name and marks, we can iterate over the sorted list of tuples, where index 0 is for the subject name, and index 1 is for the marks. Code:
Output:
Do you think there is any difference in the efficiency of dict.keys() and dict.items()? Think about how we print the key-value pairs after sorting!
The dict.items() is more efficient because after sorting, we do not need to search for the value corresponding to a given key as we have to in the case of dict.keys().
3. Sort using custom key functions
We have now covered sorting by keys based on their alphabetical order. But what if we want to custom-sort our dictionary, say by the length of the keys?
Remember, we can pass three parameters to the sorted() function - object, key, and reverse. We have only used the first parameter until now. Now is the time for the second! As mentioned before, the Key parameter is used to pass a custom function according to which we want to sort our object.
SYNTAX:
We will make use of the Lambda function, which is similar to a regular Python function but can be defined without a name. We can pass a custom lambda function to sort the keys in dict.items() by length as follows:
Remember that x[0] refers to the Subject names or the keys in the dictionary.
EXAMPLE:
To print the report card sorted by the length of Subject names, we can run the following:
Code:
Output:
Sorting by Values
Now that we have covered sorting a dictionary by keys, let us consider a scenario where we want first to sort the report card with the highest marks. (Gotta give a good first impression)
Can we sort the dictionary by values by tweaking something we have already learned? If you're thinking about the Key parameter of the sorted() function, you are right :clap:
Check out this article to learn more on Sort Dictionary by Value in Python.
SYNTAX:
Let's look back at the lambda function we created for sorting keys by length:
We mentioned that x[0] refers to the Subject names or the keys in the dictionary.
Now we can refer to the Subject marks or the values using x[1]. So now our sorted() method would look something like:
EXAMPLE:
We can run the following code to print our report card sorted by values or Subject marks:
Code:
Output:
Something doesn't look right! We wanted our marks sorted with the highest marks first, but the output is in ascending order.
This is because sorted() would sort the object in ascending order by default. This brings us to the last parameter used in the sorted() function - reverse.
Reversing the Sorted Order
SYNTAX:
To sort the object in reverse or descend order, we use the reverse parameter in the sorted() method and set it to True.
EXAMPLE: (Reverse Sort by Value)
We can run the following code to print our report card sorted by Subject marks in the reverse order:
Code:
Output:
There we go! We set the reverse keyword to True and used x[1] (Subject marks) as the key to sort the dictionary in the reverse order of values.
EXAMPLE: (Reverse Sort by Key)
We can similarly sort the report card in the reverse order of Subject names as given below. We do not need to specify the key parameter since sorted() would default sort the dictionary by keys.
Code:
Output:
Custom Sorting Algorithms
What if we have more complex sorts - say we need to custom sort our report card in a given sequence which does not follow any specific sorting order? Suppose the order is:
Maths, Science, IT, English, SST
This is neither sorted by key nor by value.
We might need to use both strings and numbers to perform such complex sorts. We would still be using our sorted() method but with some changes in the key parameter.
EXAMPLE:
The code to print the report card in our desired order is given below:
Code:
Output:
Explanation:
Looks complex? Let us break it down one by one.
- We first create a dictionary called report storing the subject names and marks.
- We then create another dictionary called order which stores the order we want to sort our dictionary. The subject name is the key, and the order in which it should appear is the value.
- In the key parameter of the sorted() method, we use order[x] as the lambda function. It sorts the order dictionary based on values (or the order in which we want the corresponding subject name to appear).
- This is a key for sorting our original report dictionary in the order we want.
- Finally, we print our report card by iterating over the sorted report dictionary.
Conclusion
- A Python dictionary stores key-value pair mappings, where the key must be unique.
- A dictionary can be sorted by keys and values using the sorted() method.
- The sorted() method takes three parameters - object, key, and reverse.
- The sorted() sorts the objects in ascending order by default. To sort the object in reverse order, we set the reverse parameter in the sorted() method to True.
- A dictionary can be sorted using pre-defined methods like dict.keys() and dict.items() as well as by custom sort functions.
- The dict.items() returns the key-value pairs of a dictionary as a list of tuples, whereas dict.keys() returns only the keys as a list.
- The dict.items() is more efficient than dict.keys() for sorting.
- We can use custom sorting algorithms to perform complex sorts, e.g., sorting by Days of week or Months of the year, with the help of both strings and numbers.