Numbers in Python
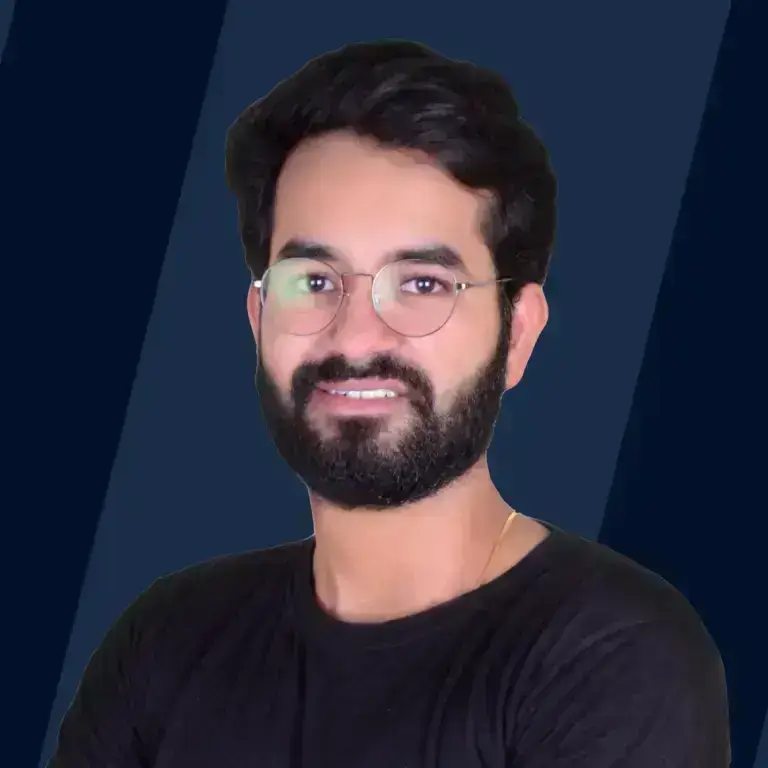
You might have used different numbers like integers and decimals in your daily activities. If you have studied mathematics till high school, you might also have learned about complex numbers. Let us see how these Python numbers can be used in a program.
In Python Numbers, there are four built-in numeric data types namely integer, floating point numbers, double, and complex numbers.
The data type of the numbers can be verified as follows:
Output:
Integer Data Type in Python
Integer data type in python numbers represents the whole numbers like 0, 1, 2, 3, 4 or negative integers such -1,-2, -3 etc.
Output:
There is no limit on values of integer data types in python.
Output:
Python also supports integer data types for other numbers such as binary, octal and hexadecimal number systems.
We can represent a binary number in Python using a 0b prefix to the number in 0b1110, hexadecimal numbers using the prefix 0x as in 0x1117, and octal numbers using the prefix 0o as in 0o1117.
Output:
Float Data Type in Python
Float represents floating point numbers in python.
Output:
In python, float data type is implemented using binary fractions. Due to this, the numbers represented using the float data type in Python are only an approximation of the actual values.
For Example:
Output:
To avoid these errors, you can perform business critical and scientific calculations using the decimal module discussed in this article.
Complex Numbers in Python
Python Numbers also supports built-in complex number data type.
Output:
As you can see in above example the data type is returned as ‘complex’.
Another way to define a complex number is by using the complex() function.
Output:
We can access the real and imaginary part of a complex number using its attributes “real” and “imag” respectively as follows.
Output:
We can also find the conjugate of the complex number using the conjugate() method.
Output:
Data Type Conversion in Python
String to Integer Conversion
We can convert a string into an integer using the int() method.
Output:
Here, the string can contain only the combination of digits from 0 to 9.
Output:
String to Float Conversion
You can transform a string into a float using the float() method.
Output:
The string given to the float() method must consist of only the decimal digits and a period i.e. decimal point (.) may be present in between them.
Output:
String to Decimal Conversion
We can also convert a string to a decimal number using the Decimal() method.
Output:
The string given to the Decimal() method must consist of only the digits, and a period may be present between them.
Output:
String to Fraction Conversion
Using the Fraction() method, you can convert a string to a fraction.
Output:
When the input string contains inappropriate characters like alphabets and punctuation marks instead of the fraction representation of a number, the Fraction() method will raise ValueError as follows.
Output:
Integer to Float Conversion
An integer can be converted into a floating point value directly using the float() method.
Output:
Float to Integer Conversion
We can also convert a floating point value to an integer using the int() method.
Output:
Float to Decimal Conversion
Although of no use, we can convert a floating point number to decimal using the Decimal() method of decimal module as follows.
Output:
Decimal to Float Conversion
We can convert the decimal number to the floating point number using the float() method of decimal module as follows.
Output:
Float to Fraction Conversion
We can convert a floating point value to a fraction using the Fraction() method.
Output:
In the above output, numerator and denominator values are very large. We can restrict the value of the numerator and denominator using the limit_denominator() method.
Output:
Fraction to Float Conversion
We can convert the fraction to the floating point number using the float() method as follows.
Output:
Fraction to Decimal Conversion
You cannot convert a fraction to a decimal value.
Output:
Decimal to Fraction Conversion
You can convert a decimal to a fraction using the Fraction() method.
Output:
Python Math Module
The math module in python contains different functions and mathematical constants that are required to perform mathematical operations. You can import the math module using the import statement as follows.
Mathematical Functions in the Math Module
There are various mathematical functions defined in the math module. Some of them are discussed below.
math.ceil()
This function rounds a floating point number to the nearest integer greater than the number.
Output:
When an integer is passed to the function as input, the output remains the same as the input number.
Output:
math.floor()
This function rounds a floating point number to the nearest integer less than the number given as input.
Output:
When an integer is passed to the function as input, the output remains the same as the input number.
Output:
math.exp()
This function takes a number, say x as input and returns ex (exponential of x) .Here e is the Euler’s number having value 2.718282.
Output:
math.factorial()
This function is used to calculate the factorial of a number as follows.
Output:
Factorial of a number is defined only for non negative integers.
Output:
Similarly, passing a negative value as input to the factorial function raises ValueError exception.
Output:
math.gcd()
The gcd() function calculates the greatest common divisor of two numbers.
Output:
Here, the input arguments must be integers.
Output:
math.log()
The log() function calculates the logarithm of a positive number to a specified base.
Output:
If there is no input given as base, the function calculates the natural log of the input number (with base e).
Output:
Logarithms are not defined for 0 and negative numbers.
Output:
math.log10()
The log10() function is used to calculate the logarithm of a positive number to the base 10.
Output:
Logarithms are not defined for 0 and negative numbers.
Output:
math.log2()
The log2() function is used to calculate the logarithm of a positive number to the base.
Output:
Logarithms are not defined for 0 and negative numbers.
Output:
math.sqrt()
The sqrt() function is used to calculate the square root of non negative numbers.
Output:
math.pow()
The pow() function is used to calculate power of one number to another.
Output:
Trigonometric Functions
The math module also offers various trigonometric functions that we can use in our programs. Some of them are discussed below.
math.sin()
This function returns sine of a number.
Output:
math.tan()
This function takes a number as input and returns the tangent of the number.
Output:
math.cos()
This function returns cosine of a number.
Output:
math.asin()
This function takes a number as input and returns its arc sine.
Output:
math.acos()
This function takes a number as input and returns its arc cosine.
Output:
math.atan()
This function takes a number as input and returns the arc tangent of the input number.
Output:
Mathematical Constants
Various mathematical constants are also defined in the math module. Following is a table of some of the mathematical constants.
Constant | Value |
---|---|
math.e | Euler’s Number (2.718281828459045) |
math.inf | Positive infinity(inf) |
math.nan | Not a Number(nan) |
math.pi | Pi (3.141592653589793) |
math.tau | Tau (6.283185307179586) |
Random Numbers in Python
If you want to generate random numbers for any use case like OTP generation or many more then you can use the random module in python for generating random numbers. You can import the module as follows.
To generate a random floating point number, you can use the random() function in the random module. The random() function generates a random floating point number when executed as shown in the example below.
Output:
To generate a random integer value, you can use the randint() function. The function takes the upper limit and lower limit of values as input arguments and returns a random integer between them as follows.
Output:
Conclusion
In this article, we have discussed built-in number data types, decimals and fractions in python. We also studied some of the functions of the math module and implemented some programs with them.