center() in Python
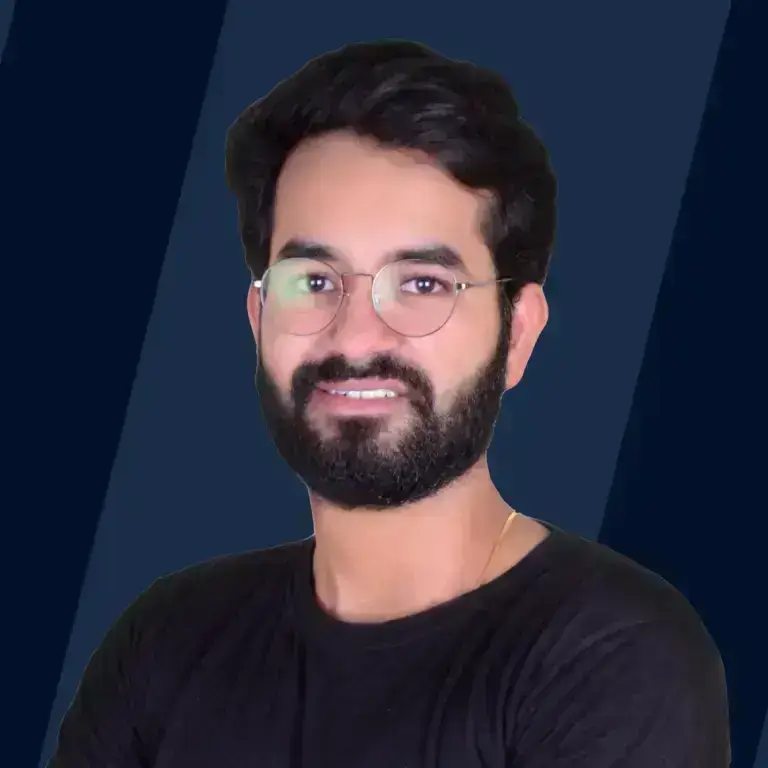
Overview
center() in Python is a method used to align text to the center of a pre-defined string length, i.e., if we want to align a 5-letter word using center() over 7 spaces, there will be one space left empty on both the sides of the word.
Syntax of Center() in Python
The syntax for center() method in Python is as follows:
str refers to the string which needs to be centered.
Parameters of center() in Python
Following are the parameter values used in the above syntax:
Width
- width is the number of blocks reserved where the string is centered. It is a required parameter for the method. It can hold any integer value ( i.e. 0 and negative integers are also considered).
Fillchar
- fillchar (optional) is the character that is padded on both sides of the centered string to fill the reserved space. By default, the value of this parameter is considered as a space.
Return Value From center() in Python
Python's center() method returns a string padded with a space or a specified character. The center() method does not alter the original string. You can save the return values in another string, use it in another expression or print it as output.
Errors While Using center() in Python
It is normal to encounter errors while using center() in Python. Following are the most common mistakes you might make while using it.
Not Specifying the Width
The width parameter is required for the center() method in Python, and omitting it will throw an error.
Output:
Padding the String with Another String
You cannot use the center() method to pad a string with another string. It will throw an error, as shown below.
Output:
Example of Center in Python
Following is an example of how the center() is used in Python:
Output:
What is Center() in Python?
When the width is smaller or equal to the length of the string, there is no need for padding or centering the string. The string will fit in the exact number of blocks as the length of the string.
While centering a string for which more blocks are reserved than its length, we will first count the number of blocks remaining for padding by subtracting the length of the string from the reserved length. i.e:
Further, use the following formula to get the number of padded blocks on both sides:
-
For Even Number of Padded Blocks:
-
For Odd Number of Padded Blocks:
Case 1: When the length of the string is odd
Case 2: When the length of the string is even
More Examples of center() in Python
Now let us understand the usage of center() in Python with a few examples as below:
center() Method With Default fillchar
The following example shows how center() in Python would be used without mentioning a default character. We will be considering 4 cases while centering a 5-letter string 'Hello':
- For a length of 1 space, i.e., as long as the string.
- For a length of 5 spaces, i.e., as long as the string.
- For a length of 10 spaces, i.e., an odd number of spaces around the string.
- For a length of 9 spaces, i.e., an even number of spaces around the string.
Output:
To understand the output better, consider the following diagram:
As shown in the above example, in example 1 of case 1, only 1 block is reserved for the 5 characters. Here, even though the other blocks aren't reserved, the word is returned as it is. Since the number of reserved blocks is less than the length of the string 'hello', there is no centering.
In example 2 again, the length of the string and the number of reserved blocks is the same. Thus, there is no visible centering. The same output would be seen in a situation where you send 0 or a negative integer to the method.
In case 2, we have reserved 10 blocks for 5 letters.
Total number of padded blocks = 10 - 5 = 5 Number of blocks padded on left = (5-1)/2 = 2 Number of blocks padded on right = (5+1)/2 = 3 Thus, the word hello is printed with 2 spaces on the left and 3 spaces on the right.
In case 3, we have reserved 11 blocks for 5 letters.
Total number of padded blocks = 11 - 5 = 6 Number of blocks padded on each side= 6/2 = 3 Thus, the word hello is printed with 3 spaces on each side.
center() in Python with a Specified Character
The following example shows how center() in Python would be used when we specify a character. Since it is only possible for a character if you want to pad an integer, make sure you place it between single or double quotation marks.
We will be considering 4 examples while centering a 5-letter string 'Hello':
- For a length of 1 space, i.e., as long as the string with a character 'a'.
- For a length of 5 spaces, i.e., as long as the string with a character ?.
- For a length of 10 spaces, i.e., an odd number of character * around the string.
- For a length of 9 spaces, i.e., an even number of characters 5 around the string.
Output:
To understand the output better, consider the following diagram:
As we can see in the above example, in example 1 of case 1, only 1 block is reserved for the 5 characters. Here, even though the other blocks aren't reserved, the word is returned as it is. So, since the number of reserved blocks is less than the length of the string hello, there is no centering. In the example 2 again, the length of the string and the number of reserved blocks is the same. Thus, there is no visible centering. In both examples, there is no need for the addition of padded characters. The same output would be seen in a situation where you send 0 or a negative integer to the method.
In case 2, we have reserved 10 blocks for 5 letters.
Total number of padded blocks = 10 - 5 = 5 Number of blocks padded on left = (5-1)/2 = 2 Number of blocks padded on right = (5+1)/2 = 3 Thus, the word hello is printed with 2 * on the left and 3 * on the right.
In case 3, we have reserved 11 blocks for 5 letters.
Total number of padded blocks = 11 - 5 = 6 Number of blocks padded on each side= 6/2 = 3 Thus, the word hello is printed with 3 5 on each side.
Conclusion
- center() in Python is used to center align a string and pad both the ends by a space or another specified character.
- center() method in Python returns a padded string. Being a returnable method, it does not alter the original string.
- Only characters and not strings can be padded at the end using Python's center() method.
- It is commonly used in improving readability and design. It is also used to pad a string with different characters of the user's choice.
See Also
Other functions that you can refer to: