How to Convert int to string in Python
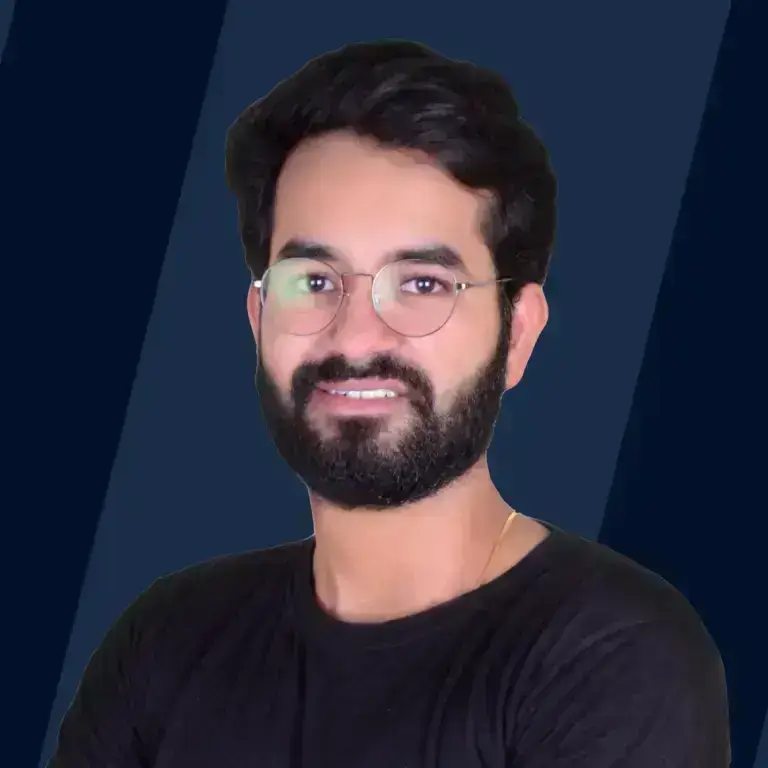
Overview
While programming in Python, we may encounter several problems requiring us to use an integer data type as a string data type, such as iterating over a number's digits or inserting an integer into a string, which is not possible using an integer data type, for that we must convert the integer into a string.
There are several methods to convert an integer into a string, like, str() function, using %s keyword, the .format() function, and f-strings.
Syntax for Converting int to string in Python.
There are four ways for converting an integer into a string in Python. The syntax for the conversions are:
str() function:
The syntax for using the str() function in python is to write the value to be typecasted inside the parenthesis.
%s keyword (argument specifiers):
The syntax for using the %s keyword is enclosing %s in quotation marks and then writing % integer after it.
.format() function:
The syntax for using the .format() function in int to string conversion is using it with an empty string and then writing the integer value inside parenthesis.
f-string:
The syntax for using the f-string method in int to string conversion is writing f and then integer value inside curly brackets enclosed in quotation marks.
Now we will learn about the working of these methods in the next section.
Converting Int to String in Python
As discussed earlier, an integer can be converted into a string using four different methods. Let us learn how these methods work:
1. str() function in Python
Python str() function (typecasting) is used to create a string version of the object (any Python data type) passed as an argument to it. The return value of the str() function is the string representation of the object. Therefore, the str() function can be used to convert an integer into a string.
2. %s keyword in Python
The %s symbol is used in Python with a large variety of data types. This symbol is mainly used for the concatenation of strings together while allowing us to format values inside the string. We can use the %s keyword to convert an integer into a string as it automatically provides type conversion from value to a string.
3. .format() function in Python
The string format() method is primarily used for formatting the specified values and converting the result as a string. The format() function always returns the inputted value as a string data type, no matter the input data type, as the formatted value is inserted in the placeholders "{}" in {}.format(value), where "{}" is a part of a string. Using the string format() method we can easily convert integers into strings.
4. f-string in Python
Literal String Interpolation is also known as f-strings (because of the f character written before the formatted string). The main idea behind f-strings is to make string interpolation (the concatenating and formatting of strings) simpler. The string can also be formatted like in the string format() method. This is used to insert python expressions inside strings (by evaluating the expression and then inserting it after conversion to a string), using the f-strings method, we can insert integers into strings, thus, converting them to a string.
Out of all these methods, str() function shoud be preferred as it is the best practice for value to string conversion if formatting is not involved, as the str() function is solely used for specifiying the data type of a value as a string. This makes the code readable and easy to understand.
We will now look at the examples for each method in the next section, for a clear understanding of how these ways work.
Example of Int to String Conversion in Python
Examples for int to string conversion using different methods:
Using str() function in Python
Let us look at an example of converting an integer into a string using the str() function (typecasting).
Code:
Output:
Explanation:
In the above example, we are first initializing an integer num, then on checking its data type, the output shows the data type is int. We then convert the value into a string using the str() function. The result can be seen in the output that the data type after conversion is str.
Using %s keyword in Python
Let us look at an example of converting an integer into a string using the %s keyword.
Code:
Output:
Explanation:
In the above example, we are checking the data type of num, which shows the data type is int. We then convert the value into a string using the %s keyword in Python. The result can be seen in the output that the data type after conversion is str.
Using .format() function in Python
Let us look at an example of converting an integer into a string using the .format() function.
Code:
Output:
Explanation:
In the above example, we are checking the data type of num, which shows the data type is int. We then convert the value into a string using the .format() function in Python. The result can be seen in the output that the data type after conversion is str.
Using f-strings in Python
Let us look at an example of converting an integer into a string using f-strings.
Code:
Output:
Explanation:
In the above example, we are checking the data type of num, which shows the data type is int. We then convert the value into a string using the f-strings in Python. The result can be seen in the output that the data type after conversion is str.
Ready to become a Python-certified professional? Our Python online course offers comprehensive training and a path to certification. Enroll today!
Conclusion
- There are several methods for converting an integer into a string, we can use typecasting (str() function), %s keyword, .format() function and f-strings method.
- While using the .format() function and f-strings method, we can also format the representation of values while converting the value into a string.
- Although, there are four ways to convert an integer into a string, the str() function (typecasting) is the most practical method for type conversion to string.