F String in Python
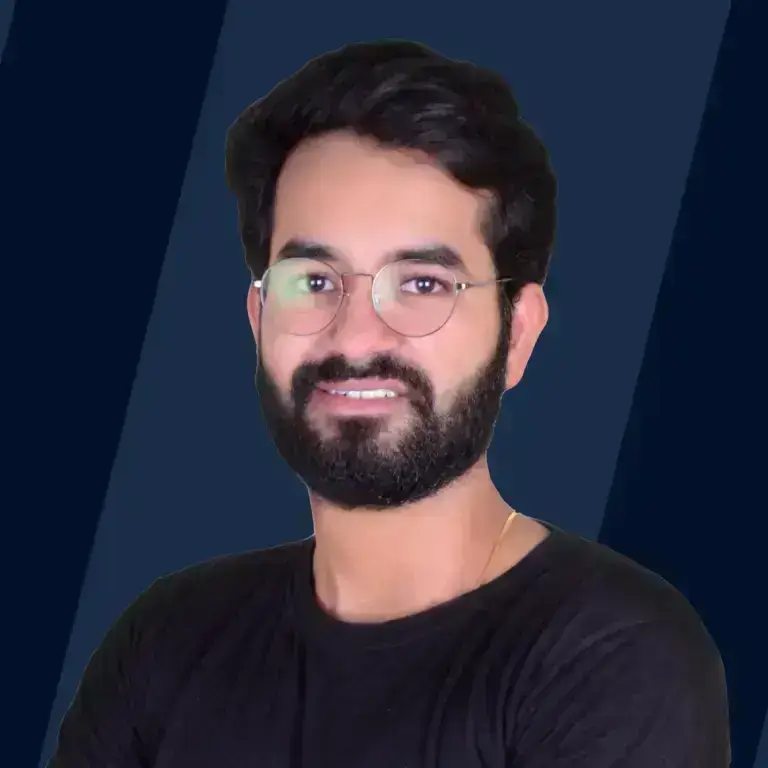
F String in Python, also known as a formatted string literal is a concept introduced in Python 3.6 that is used in creating user-friendly strings. They are used for a variety of purposes like alignment of strings, evaluation of expressions, etc. f-strings are evaluated at runtime making them highly powerful.
Example of f-string in Python
While writing f-string in Python, we prefix the string with an f (or F). The string can be created using single quotes, double quotes, or triple quotes. The expression to be evaluated within the f-string is placed in curly braces. The content of the curly braces can be anything, depending on the situation.
Now, let's understand f-string in Python in a better way using an example.
Output:
In the above example, we used a variable val which contained the value Apple. In the print statement, we saw how using f string in Python, we added the word Apple at different parts of the string.
The best and most interesting part about f-string in Python is that it evaluates expressions at runtime, which makes it more efficient than the other string formatting methods. In the following example, we can see how the f-string is used to calculate the product of 2 values at runtime:
Output:
More Examples of f-strings in Python
f-string in Python is very useful and efficient in various situations. Let's consider the vast number of usages of f-string in Python through examples.
f-string in Python Used with the Class Object
In Python, the f-string can be used with class objects. Each class has 2 distinct methods that can be used to convert an object to a string. These are as follows:
- __repr__(): It produces a more descriptive string that may provide debug information.
- __str__(): It produces a string that is both displayable and comprehensible to the user. That is, it should not include any confidential information, nor should it contain any debug messages. f-string in Python automatically calls the __str__() method on the object you supply it with.
To understand f-string in Python better, let's consider an example of a Student class that stores names and roll number of students.
Output:
F-string in Dictionary
Let's consider a situation where we want to evaluate a dictionary. Using fstring in Python, it can be done in the following manner:
Output:
Python f-string Debug
Using the self-documenting expression represented by the = character, f-string in Python can be used for debugging. Consider the below example:
Output:
In the above example, you can see how the = character is used to debug the expression. When using fstring for debugging, we see that the output fstring is enclosed in single quotes. This is because in this situation, the repr of the variable is used instead of its value.
Let's see the usage of f-string in debugging using another example.
Output:
The above example uses the tan function in debug mode.
Python Multiline f-string
We have already seen how we can use f-string while formatting single-line strings. Even multiline strings can be formatted using f-string in Python. To do so, we enclose the f-string in round brackets and each of the component strings is preceded by the f character.
Example 1:
Output:
That's not the only way we can print multiline strings using f-string in Python. In the next 2 examples we can see how backslash and triple-double quotation marks can be used to achieve the same:
Example 2:
Output:
Example 3:
Output:
Python f-string Calling Function
We can also call functions in f-string. Consider the following example:
Output:
Python f-string Escaping Characters
While writing f-string in Python, we make use of special characters like quotes and curly brackets. What if we want it to be a part of the string? How would the Python online compiler differentiate between the f-string syntax and value? For this purpose, we make use of escape characters in f-string.
To escape a curly bracket, we double the character. While a single quote is escaped using a backslash.
Example:
Output:
We can display more braces if we use more than triple braces.
Example:
Output:
Python f-string Format Datetime
Date and time can be formatted in various ways. In Python, f-string helps you achieve this seamlessly.
Example:
Output:
The above example displays a formatted current DateTime. In the first line, we see the date in YYYY-MM-DD format separated by a hyphen (-). Here, only the hour and minutes are mentioned in the time. In the second line, we displayed the date in DD/MM/YY format separating the individual elements of the date using a /. The time contains hours, minutes, and seconds in this example.
Python f-string Format Floats
Floating-point values in Python can be displayed in various ways - with different positioning of the decimal point. f-string in Python helps us specify the precision of the floating-point value and display it accordingly.
Example:
Output:
In the above example, we can see the value of Pi being printed with 2 and 5 digits after the decimal point respectively.
Python f-string Format Width
We can also use f-string in Python to decide the width of the value that has to be displayed. The width specifier is used for this. It determines the size of the value. If the value is less than the set width, it may be filled with spaces or other specified characters.
Example:
Output:
The example prints three columns and 12 rows. The columns display tables of 1, 12 and 24 respectively. The first column uses 0 to fill values having a width less than 2. The other 2 columns have a width of 3 and 4 respectively and use space to fill the width.
Python f-string Justify String
By default, strings in Python are justified to the left. To justify the strings to the right, we may use the > character. The colon character is followed by the > character.
Suppose we have to fit the word hello in 5, 10 and 15 blocks respectively.
Output:
The first line shows the index of each block. When the word 'hello' is right-justified in the strings such that they are to fit 5, 10, and 15 blocks respectively, we see the output as shown in the above example.
Python f-string Numeric Notations
f-string in Python can be also used to represent numbers in various notations like hexadecimal, octal, etc. Let's consider the examples below that is used to print the number 786 in various formats as mentioned in the comments:
Output:
Python f-string Quotation Marks
To use quotation marks in f-string in Python, you have to only avoid using the same type of quotations as used with the f. And in case you have to use the same quotes in the string as well as with the f, use the backslash character to escape.
Let's understand this using the below example.
Output:
Speed
The rationale for using this formatting style is that it is quick. Rather than using constant values, the f-string evaluates at runtime. It uses a simple syntax to incorporate expressions into string literals. It is efficient since it evaluates at runtime rather than using a constant value.
Let's compare the speed of execution with and without f-string. For this, we will use the time it function to check how much time it takes to evaluate the f-string. The value is variable and changes at each execution.
Example:
Output:
As we see in the above example, f-string in Python executes much faster than normal strings.
Backslash
Backslash escapes can be used in the string component of an f-string, but cannot be used to escape in the expression section of an f-string.
Example:
Output:
Inline Comments
The # sign cannot be used in the string inside the f-string. It will generate a syntax error.
Example:
Output:
Conclusion
- Strings can be formatted using the format() method, ``% operatororf-string` in Python.
- We may use any of the three methods, but the f-string technique is more succinct, readable, and convenient.
- In this article, we learned how to use f-Strings to display variable values, evaluate expressions, call methods on other Python objects, and call Python functions.