Reduce Function in Python
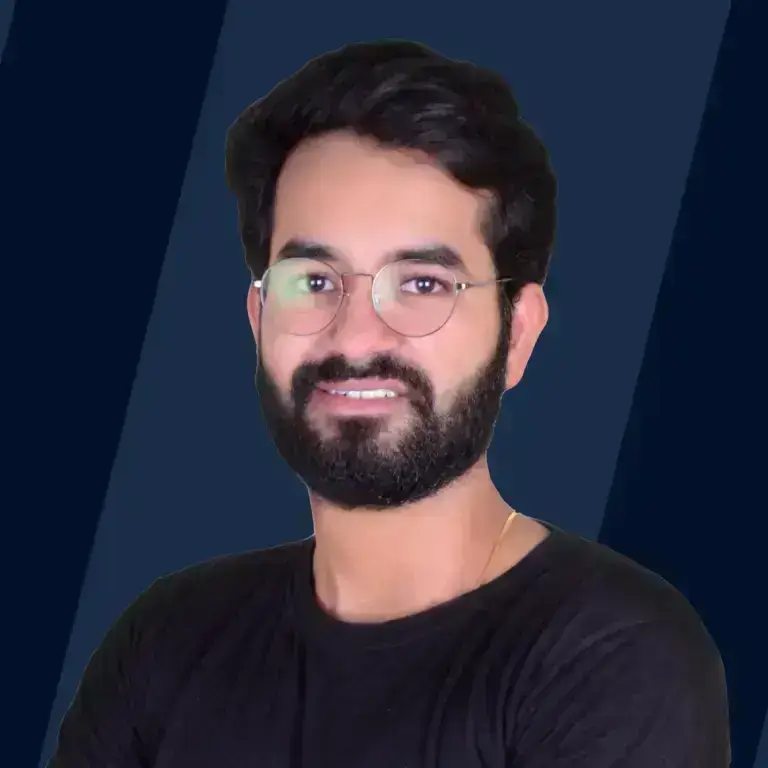
Introduction to reduce() in Python
reduce in Python from the functools module is a powerful tool for performing functional computations. This function takes a predefined function and an iterable (like a list, tuple, or dictionary) and methodically applies the function across the iterable to produce a single output value.
Unlike a traditional for loop, which requires explicit programming, reduce() simplifies this process, efficiently reducing the iterable to a single result—whether it be an integer, string, or boolean. This function, programmed in C, offers optimized performance, making it an effective choice for data processing. By leveraging reduce(), developers can execute complex computations with minimal code, ensuring both code efficiency and readability in Python applications.
How Python reduce() Function works
reduce in Python is a part of the functools module and doesn't return multiple values; it just returns a single value.
How the Reduce Function Operates in Python:
- Initially, the specified function is utilized on the initial pair of elements in the iterable.
- Subsequently, the same function is employed on the outcome of the first operation and the subsequent element in the iterable.
- This procedure is repeated sequentially through the entire iterable.
- Finally, a solitary value, derived from executing the reduce function on the entire iterable, is produced as the output.
Examples of Reduce Function in Python
Using a lambda function
In this example, we have defined a lambda function that calculates the sum of two numbers on the passed list as an iterable using the reduce function.
Code:
Output:
The above python program returns the sum of the whole list as a single value result which is calculated by applying the lambda function, which solves the equation (((1+2)+3)+4).
Using a pre-defined function
Now, a pre-defined function, product, which returns the product of 2 numbers passed into it, is used as an argument in the reduce function, which is passed along with a list of numbers.
Code:
Output:
We get the result as 210 because the steps followed by the reduce function calculate the result of the following equation(((2x5)x3)x7). First, the multiplication of 2 and 5 is done, and the result of this multiplication is further done with all the other elements of the list one by one.
Using Operator Functions
Sometimes reduce function is implemented along with Operator functions in Python. Operator functions are the basic pre-defined mathematical, logical, and bitwise operations combined inside a single module in Python named operator. Let's explore how to reduce functions is used with operator functions.
Code:
Output:
Applying Python Reduce to an Empty List
You must wonder what will happen if an empty list is passed to the reduce function. Let's check it with the help of an example.
Code:
Output:
So we got a Type Error stating that there is no initial value for an empty sequence. This means that in the case of an empty list, a third argument called initializer has to be passed in the reduce function.
Reducing Iterables With Python’s reduce()
Summing Numeric Values
Python's reduce() function, found in the functools module, is adept at summing numeric values in an iterable, such as a list. This function applies a given function cumulatively to the items of the iterable, producing a single result.
Code:
Output:
Multiplying Numeric Values
Similarly, Python's reduce() function can be utilized for multiplying numeric values within an iterable. By defining a multiplication function and passing it to reduce() along with an iterable like a list, we can calculate the product of all elements in the list.
Code:
Output:
Finding the Minimum and Maximum Value
reduce in Python can calculate Minimum and Maximum values from the iterable by comparing items within the given iterable. The below program includes two different pre-defined functions.
The first function takes two arguments, a and b, and return their minimum, while the second function will use a similar process to return the maximum value.
Code:
Output:
Learn from industry experts and get certified in Python programming. Enroll in our Python course and boost your professional credibility.
Checking if All Values Are True
To check whether all values in the given iterable are True or not, we can write a function that can takes two arguments and returns True if both arguments are true. If one or both arguments are false, the function will return False.
Code:
Output:
Checking if Any Value Is True
To check whether any value in the given iterable is True or not, we can write a function that takes two arguments and returns True if either one of the two arguments is true. If both arguments are false, then the function will return False.
Code:
Output:
Difference Between Map and Reduce
After learning about Reduce, you might get confused between the reducing and map functions. The main difference between these two is that map() in python is applied to every item of an iterable one at the time, and in a result an iterator is returned.
Lets see how is a map() function implemented
Code:
Output:
In the above program, the map function applies the lambda function to every element in the iterable one by one, and at the end, an iterator is returned. Here, the type of returned list is <class 'map'>.
Reduce() vs Accumulate()
In the itertools module, a function named accumulate() is present, which takes an iterable as an argument. Sometimes, a function is also passed as an optional second argument.
Syntax : itertools.accumulate(iterable)
The accumulate function returns an iterator that consists of accumulated sums of every item in the iterable. So the working of accumulate varies from the reduce function where only a single value is the result.
Code:
Output:
As we can see, the return type is of <class 'itertools.accumulate'> and the iterator returned is a result of the accumulated sums expression [1, (1+2), (1+2+3), (1+2+3+4)].
Reduce vs Python For Loop
A For loop can do the same thing that a reduce function does in Python, so there is no difference in the functionality between these two, but when it comes to the amount of code to be written to implement the same thing, reduce function has a big advantage over traditional python for loop. Let's understand with an example.
Code:
Output:
The above program calculates the sum of all elements present in the nums array. Both for loop and reduce functions produce the same correct output. The only difference between the traditional loop and the reduce function is that the latter method outperforms them when the list size is very large, as it is optimized and efficient.
Reduce vs Python List Comprehension
Reduce in python returns a single value as a result, while a list comprehension when applied to a list, returns another list.
Sometimes, like flattening a list of lists into a single list, we can use list comprehension. Let's deep dive into this flattening list with the help of an example.
Code:
Output:
In the above program, a 2D list is flattened by using the reduce function, where the first two lists are combined and a new list is created, which is further passed to the reduce function along with the successive list.
In the case of list comprehension, iteration is done for every row in the matrix, and every item in that particular row is returned. Use this Python Online Compiler to compile your code.
Reduce vs Itertools.accumulate
Python offers two similar yet distinct functions for aggregating values in iterables: reduce() from the functools module and accumulate() from the itertools module. Both are used for performing computations over a list or other iterables, but they differ in their operation and output.
Code Example with reduce():
Code Example with accumulate():
In summary, while both reduce() and accumulate() can be used for aggregating values, the choice depends on whether you need just the final result or the entire sequence of intermediate results. reduce() is ideal for a single output, whereas accumulate() offers more insight into the aggregation process.
reduce() function with three parameters
As we have already covered a case where an empty list was passed as an argument to the reduce in Python, a new 3rd argument initializer is used.
reduce() places the 3rd parameter before the value of the second one if it’s present. Thus, if the 2nd argument is an empty sequence, then the 3rd argument serves as the default one.
Code:
Output:
The above program takes initializer = 3 as an argument in the reduce function, and the lambda function calculates the sum of the whole tuple. Still, in this case, the sum will be initialized by the default value of 3, which is the value of the initializer. Therefore the output will be sum of elements in tuple + 3 = 56
Conclusion
- In Python, the reduce() function enables reduction operations on iterables, utilizing both Python callables and lambda functions. This function systematically applies a specified function to the elements in an iterable, condensing them into a single, cumulative value.
- Python's reduce function performs various common problems like addition or multiplication of numbers in iterables.
- reduce in Python is a very popular method in functional programming even though it has been replaced by many Pythonic functions like sum(), any(), min() etc.
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.